Chapter 3 : The 8051 Instruction Set
- 3.1 Types of instructions
- 3.2 Description of the 8051 instructions
Introduction
The process of writing program for the microcontroller mainly consists of giving instructions (commands) in the specific order in which they should be executed in order to carry out a specific task. As electronics cannot “understand” what for example an instruction “if the push button is pressed- turn the light on” means, then a certain number of simpler and precisely defined orders that decoder can recognise must be used. All commands are known as INSTRUCTION SET. All microcontrollers compatibile with the 8051 have in total of 255 instructions, i.e. 255 different words available for program writing.
At first sight, it is imposing number of odd signs that must be known by heart. However, It is not so complicated as it looks like. Many instructions are considered to be “different”, even though they perform the same operation, so there are only 111 truly different commands. For example: ADD A,R0, ADD A,R1, ... ADD A,R7 are instructions that perform the same operation (additon of the accumulator and register). Since there are 8 such registers, each instruction is counted separately. Taking into account that all instructions perform only 53 operations (addition, subtraction, copy etc.) and most of them are rarely used in practice, there are actually 20-30 abbreviations to be learned, which is acceptable.
3.1 Types of instructions
Depending on operation they perform, all instructions are divided in several groups:
- Arithmetic Instructions
- Branch Instructions
- Data Transfer Instructions
- Logic Instructions
- Bit-oriented Instructions
The first part of each instruction, called MNEMONIC refers to the operation an instruction performs (copy, addition, logic operation etc.). Mnemonics are abbreviations of the name of operation being executed. For example:
INC R1
- Means: Increment register R1 (increment register R1);LJMP LAB5
- Means: Long Jump LAB5 (long jump to the address marked as LAB5);JNZ LOOP
- Means: Jump if Not Zero LOOP (if the number in the accumulator is not 0, jump to the address marked as LOOP);
The other part of instruction, called OPERAND is separated from mnemonic by at least one whitespace and defines data being processed by instructions. Some of the instructions have no operand, while some of them have one, two or three. If there is more than one operand in an instruction, they are separated by a comma. For example:
RET
- return from a subroutine;JZ TEMP
- if the number in the accumulator is not 0, jump to the address marked as TEMP;ADD A,R3
- add R3 and accumulator;CJNE A,#20,LOOP
- compare accumulator with 20. If they are not equal, jump to the address marked as LOOP;
Arithmetic instructions
Arithmetic instructions perform several basic operations such as addition, subtraction, division, multiplication etc. After execution, the result is stored in the first operand. For example:
ADD A,R1
- The result of addition (A+R1) will be stored in the accumulator.ARITHMETIC INSTRUCTIONS | |||
---|---|---|---|
Mnemonic | Description | Byte | Cycle |
ADD A,Rn | Adds the register to the accumulator | 1 | 1 |
ADD A,direct | Adds the direct byte to the accumulator | 2 | 2 |
ADD A,@Ri | Adds the indirect RAM to the accumulator | 1 | 2 |
ADD A,#data | Adds the immediate data to the accumulator | 2 | 2 |
ADDC A,Rn | Adds the register to the accumulator with a carry flag | 1 | 1 |
ADDC A,direct | Adds the direct byte to the accumulator with a carry flag | 2 | 2 |
ADDC A,@Ri | Adds the indirect RAM to the accumulator with a carry flag | 1 | 2 |
ADDC A,#data | Adds the immediate data to the accumulator with a carry flag | 2 | 2 |
SUBB A,Rn | Subtracts the register from the accumulator with a borrow | 1 | 1 |
SUBB A,direct | Subtracts the direct byte from the accumulator with a borrow | 2 | 2 |
SUBB A,@Ri | Subtracts the indirect RAM from the accumulator with a borrow | 1 | 2 |
SUBB A,#data | Subtracts the immediate data from the accumulator with a borrow | 2 | 2 |
INC A | Increments the accumulator by 1 | 1 | 1 |
INC Rn | Increments the register by 1 | 1 | 2 |
INC Rx | Increments the direct byte by 1 | 2 | 3 |
INC @Ri | Increments the indirect RAM by 1 | 1 | 3 |
DEC A | Decrements the accumulator by 1 | 1 | 1 |
DEC Rn | Decrements the register by 1 | 1 | 1 |
DEC Rx | Decrements the direct byte by 1 | 1 | 2 |
DEC @Ri | Decrements the indirect RAM by 1 | 2 | 3 |
INC DPTR | Increments the Data Pointer by 1 | 1 | 3 |
MUL AB | Multiplies A and B | 1 | 5 |
DIV AB | Divides A by B | 1 | 5 |
DA A | Decimal adjustment of the accumulator according to BCD code | 1 | 1 |
Branch Instructions
There are two kinds of branch instructions:
Unconditional jump instructions: upon their execution a jump to a new location from where the program continues execution is executed.
Conditional jump instructions: a jump to a new program location is executed only if a specified condition is met. Otherwise, the program normally proceeds with the next instruction.
BRANCH INSTRUCTIONS | |||
---|---|---|---|
Mnemonic | Description | Byte | Cycle |
ACALL addr11 | Absolute subroutine call | 2 | 6 |
LCALL addr16 | Long subroutine call | 3 | 6 |
RET | Returns from subroutine | 1 | 4 |
RETI | Returns from interrupt subroutine | 1 | 4 |
AJMP addr11 | Absolute jump | 2 | 3 |
LJMP addr16 | Long jump | 3 | 4 |
SJMP rel | Short jump (from –128 to +127 locations relative to the following instruction) | 2 | 3 |
JC rel | Jump if carry flag is set. Short jump. | 2 | 3 |
JNC rel | Jump if carry flag is not set. Short jump. | 2 | 3 |
JB bit,rel | Jump if direct bit is set. Short jump. | 3 | 4 |
JBC bit,rel | Jump if direct bit is set and clears bit. Short jump. | 3 | 4 |
JMP @A+DPTR | Jump indirect relative to the DPTR | 1 | 2 |
JZ rel | Jump if the accumulator is zero. Short jump. | 2 | 3 |
JNZ rel | Jump if the accumulator is not zero. Short jump. | 2 | 3 |
CJNE A,direct,rel | Compares direct byte to the accumulator and jumps if not equal. Short jump. | 3 | 4 |
CJNE A,#data,rel | Compares immediate data to the accumulator and jumps if not equal. Short jump. | 3 | 4 |
CJNE Rn,#data,rel | Compares immediate data to the register and jumps if not equal. Short jump. | 3 | 4 |
CJNE @Ri,#data,rel | Compares immediate data to indirect register and jumps if not equal. Short jump. | 3 | 4 |
DJNZ Rn,rel | Decrements register and jumps if not 0. Short jump. | 2 | 3 |
DJNZ Rx,rel | Decrements direct byte and jump if not 0. Short jump. | 3 | 4 |
NOP | No operation | 1 | 1 |
Data Transfer Instructions
Data transfer instructions move the content of one register to another. The register the content of which is moved remains unchanged. If they have the suffix “X” (MOVX), the data is exchanged with external memory.
DATA TRANSFER INSTRUCTIONS | |||
---|---|---|---|
Mnemonic | Description | Byte | Cycle |
MOV A,Rn | Moves the register to the accumulator | 1 | 1 |
MOV A,direct | Moves the direct byte to the accumulator | 2 | 2 |
MOV A,@Ri | Moves the indirect RAM to the accumulator | 1 | 2 |
MOV A,#data | Moves the immediate data to the accumulator | 2 | 2 |
MOV Rn,A | Moves the accumulator to the register | 1 | 2 |
MOV Rn,direct | Moves the direct byte to the register | 2 | 4 |
MOV Rn,#data | Moves the immediate data to the register | 2 | 2 |
MOV direct,A | Moves the accumulator to the direct byte | 2 | 3 |
MOV direct,Rn | Moves the register to the direct byte | 2 | 3 |
MOV direct,direct | Moves the direct byte to the direct byte | 3 | 4 |
MOV direct,@Ri | Moves the indirect RAM to the direct byte | 2 | 4 |
MOV direct,#data | Moves the immediate data to the direct byte | 3 | 3 |
MOV @Ri,A | Moves the accumulator to the indirect RAM | 1 | 3 |
MOV @Ri,direct | Moves the direct byte to the indirect RAM | 2 | 5 |
MOV @Ri,#data | Moves the immediate data to the indirect RAM | 2 | 3 |
MOV DPTR,#data | Moves a 16-bit data to the data pointer | 3 | 3 |
MOVC A,@A+DPTR | Moves the code byte relative to the DPTR to the accumulator (address=A+DPTR) | 1 | 3 |
MOVC A,@A+PC | Moves the code byte relative to the PC to the accumulator (address=A+PC) | 1 | 3 |
MOVX A,@Ri | Moves the external RAM (8-bit address) to the accumulator | 1 | 3-10 |
MOVX A,@DPTR | Moves the external RAM (16-bit address) to the accumulator | 1 | 3-10 |
MOVX @Ri,A | Moves the accumulator to the external RAM (8-bit address) | 1 | 4-11 |
MOVX @DPTR,A | Moves the accumulator to the external RAM (16-bit address) | 1 | 4-11 |
PUSH direct | Pushes the direct byte onto the stack | 2 | 4 |
POP direct | Pops the direct byte from the stack/td> | 2 | 3 |
XCH A,Rn | Exchanges the register with the accumulator | 1 | 2 |
XCH A,direct | Exchanges the direct byte with the accumulator | 2 | 3 |
XCH A,@Ri | Exchanges the indirect RAM with the accumulator | 1 | 3 |
XCHD A,@Ri | Exchanges the low-order nibble indirect RAM with the accumulator | 1 | 3 |
Logic Instructions
Logic instructions perform logic operations upon corresponding bits of two registers. After execution, the result is stored in the first operand.
LOGIC INSTRUCTIONS | |||
---|---|---|---|
Mnemonic | Description | Byte | Cycle |
ANL A,Rn | AND register to accumulator | 1 | 1 |
ANL A,direct | AND direct byte to accumulator | 2 | 2 |
ANL A,@Ri | AND indirect RAM to accumulator | 1 | 2 |
ANL A,#data | AND immediate data to accumulator | 2 | 2 |
ANL direct,A | AND accumulator to direct byte | 2 | 3 |
ANL direct,#data | AND immediae data to direct register | 3 | 4 |
ORL A,Rn | OR register to accumulator | 1 | 1 |
ORL A,direct | OR direct byte to accumulator | 2 | 2 |
ORL A,@Ri | OR indirect RAM to accumulator | 1 | 2 |
ORL direct,A | OR accumulator to direct byte | 2 | 3 |
ORL direct,#data | OR immediate data to direct byte | 3 | 4 |
XRL A,Rn | Exclusive OR register to accumulator | 1 | 1 |
XRL A,direct | Exclusive OR direct byte to accumulator | 2 | 2 |
XRL A,@Ri | Exclusive OR indirect RAM to accumulator | 1 | 2 |
XRL A,#data | Exclusive OR immediate data to accumulator | 2 | 2 |
XRL direct,A | Exclusive OR accumulator to direct byte | 2 | 3 |
XORL direct,#data | Exclusive OR immediate data to direct byte | 3 | 4 |
CLR A | Clears the accumulator | 1 | 1 |
CPL A | Complements the accumulator (1=0, 0=1) | 1 | 1 |
SWAP A | Swaps nibbles within the accumulator | 1 | 1 |
RL A | Rotates bits in the accumulator left | 1 | 1 |
RLC A | Rotates bits in the accumulator left through carry | 1 | 1 |
RR A | Rotates bits in the accumulator right | 1 | 1 |
RRC A | Rotates bits in the accumulator right through carry | 1 | 1 |
Bit-oriented Instructions
Similar to logic instructions, bit-oriented instructions perform logic operations. The difference is that these are performed upon single bits.
BIT-ORIENTED INSTRUCTIONS | |||
---|---|---|---|
Mnemonic | Description | Byte | Cycle |
CLR C | Clears the carry flag | 1 | 1 |
CLR bit | Clears the direct bit | 2 | 3 |
SETB C | Sets the carry flag | 1 | 1 |
SETB bit | Sets the direct bit | 2 | 3 |
CPL C | Complements the carry flag | 1 | 1 |
CPL bit | Complements the direct bit | 2 | 3 |
ANL C,bit | AND direct bit to the carry flag | 2 | 2 |
ANL C,/bit | AND complements of direct bit to the carry flag | 2 | 2 |
ORL C,bit | OR direct bit to the carry flag | 2 | 2 |
ORL C,/bit | OR complements of direct bit to the carry flag | 2 | 2 |
MOV C,bit | Moves the direct bit to the carry flag | 2 | 2 |
MOV bit,C | Moves the carry flag to the direct bit | 2 | 3 |
3.2 Description of all 8051 instructions
Here is a list of the operands and their meanings:
- A - accumulator;
Rn - is one of working registers (R0-R7) in the currently active RAM memory bank; - Direct - is any 8-bit address register of RAM. It can be any general-purpose register or a SFR (I/O port, control register etc.);
- @Ri - is indirect internal or external RAM location addressed by register R0 or R1;
- #data - is an 8-bit constant included in instruction (0-255);
- #data16 - is a 16-bit constant included as bytes 2 and 3 in instruction (0-65535);
- addr16 - is a 16-bit address. May be anywhere within 64KB of program memory;
- addr11 - is an 11-bit address. May be within the same 2KB page of program memory as the first byte of the following instruction;
- rel - is the address of a close memory location (from -128 to +127 relative to the first byte of the following instruction). On the basis of it, assembler computes the value to add or subtract from the number currently stored in the program counter;
- bit - is any bit-addressable I/O pin, control or status bit; and
- C - is carry flag of the status register (register PSW).
ACALL addr11 - Absolute subroutine call
addr11: Subroutine address
Description: Instruction unconditionally calls a subroutine located at the specified code address. Therefore, the current address and the address of called subroutine must be within the same 2K byte block of the program memory, starting from the first byte of the instruction following ACALL.
Syntax: ACALL [subroutine name];
Bytes: 2 (instruction code, subroutine address);
STATUS register flags: No flags are affected.
Bytes: 2 (instruction code, subroutine address);
STATUS register flags: No flags are affected.
EXAMPLE:
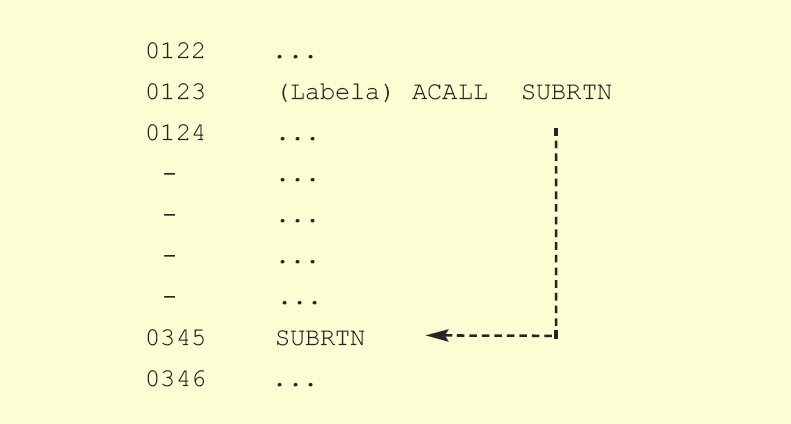
Before execution: PC=0123h
After execution: PC=0345h
After execution: PC=0345h
ADD A,Rn - Adds the register Rn to the accumulator
A: accumulator
Rn: any R register (R0-R7)
Rn: any R register (R0-R7)
Description: Instruction adds the register Rn (R0-R7) to the accumulator. After addition, the result is stored in the accumulator.
Syntax: ADD A,Rn;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
EXAMPLE:
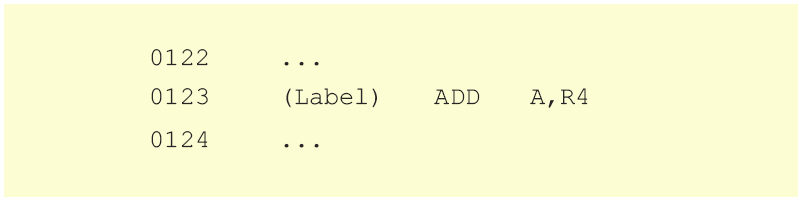
Before execution: A=2Eh (46 dec.) R4=12h (18 dec.)
After execution: A=40h (64 dec.) R4=12h
After execution: A=40h (64 dec.) R4=12h
ADD A,@Ri - Adds the indirect RAM to the accumulator
A: accumulator
Ri: Register R0 or R1
Ri: Register R0 or R1
Description: Instruction adds the indirect RAM to the accumulator. Address of indirect RAM is stored in the Ri register (R0 or R1). After addition, the result is stored in the accumulator.
Syntax: ADD A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
EXAMPLE:
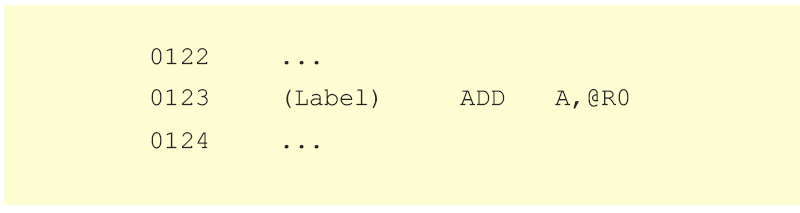
Register address: SUM = 4Fh R0=4Fh
Before execution: A= 16h (22 dec.) SUM= 33h (51 dec.)
After execution : A= 49h (73 dec.)
Before execution: A= 16h (22 dec.) SUM= 33h (51 dec.)
After execution : A= 49h (73 dec.)
ADD A,direct - Adds the direct byte to the accumulator
A: accumulator
Direct: Arbitrary register with address 0 - 255 (0 - FFh)
Direct: Arbitrary register with address 0 - 255 (0 - FFh)
Description: Instruction adds the direct byte to the accumulator. As it is direct addressing, the direct can be any SFR or general-purpose register with address 0-7 Fh. The result is stored in the accumulator.
Syntax: ADD A, register name;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: C, OV and AC;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: C, OV and AC;
EXAMPLE:
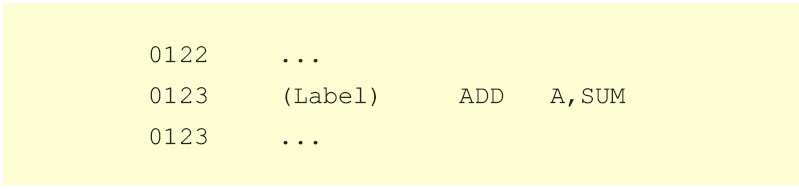
Before execution: SUM= 33h (51 dec.) A= 16h (22 dec.)
After execution: SUM= 33h (73 dec.) A= 49h (73 dec.)
After execution: SUM= 33h (73 dec.) A= 49h (73 dec.)
ADDC A,Rn - Adds the register to the accumulator with a carry flag
A: accumulator
Rn: any R register (R0-R7)
Rn: any R register (R0-R7)
Description: Instruction adds the accumulator with a carry flag and Rn register (R0-R7). After addition, the result is stored in the accumulator.
Syntax: ADDC A,Rn;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
EXAMPLE:

Before execution: A= C3h (195 dec.) R0= AAh (170 dec.) C=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
ADD A,#data - Adds the immediate data to the accumulator
A: accumulator
Data: constant within 0-255 (0-FFh)
Data: constant within 0-255 (0-FFh)
Description: Instruction adds data (0-255) to the accumulator. After addition, the result is stored in the accumulator.
Syntax: ADD A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV and AC;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV and AC;
EXAMPLE:

Before execution: A= 16h (22 dec.)
After execution: A= 49h (73 dec.)
After execution: A= 49h (73 dec.)
ADDC A,direct - Adds the direct byte to the acumulator with a carry flag
A: accumulator
Direct: arbitrary register with address 0-255 (0-FFh)
Direct: arbitrary register with address 0-255 (0-FFh)
Description: Instruction adds the direct byte to the accumulator with a carry flag. As it is direct addressing, the register can be any SFRs or general purpose register with address 0-7Fh (0-127dec.). The result is stored in the accumulator.
Syntax: ADDC A, register address;
Bytes: 2 (instruction code, direct);
STATUS register flags: C, OV and AC;
Bytes: 2 (instruction code, direct);
STATUS register flags: C, OV and AC;
EXAMPLE:

Before execution: A= C3h (195 dec.) TEMP = AAh (170 dec.) C=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
ADDC A,@Ri - Adds the indirect RAM to the accumulator with a carry flag
A: accumulator
Ri: Register R0 or R1
Ri: Register R0 or R1
Description: Instruction adds the indirect RAM to the accumulator with a carry flag. RAM address is stored in the Ri register (R0 or R1). After addition, the result is stored in the accumulator.
Syntax: ADDC A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV and AC;
EXAMPLE:

Register address: SUM = 4Fh R0=4Fh
Before execution: A= C3h (195 dec.) SUM = AAh (170 dec.) C=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
Before execution: A= C3h (195 dec.) SUM = AAh (170 dec.) C=1
After execution: A= 6Eh (110 dec.) AC=0, C=1, OV=1
ADDC A,#data - Adds the immediate data to the accumulator with a carry flag
A: accumulator
Data: constant with address 0-255 (0-FFh)
Data: constant with address 0-255 (0-FFh)
Description: Instruction adds data (0-255) to the accumulator with a carry flag. After addition, the result is stored in the accumulator.
Syntax: ADDC A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV and AC;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV and AC;
EXAMPLE:
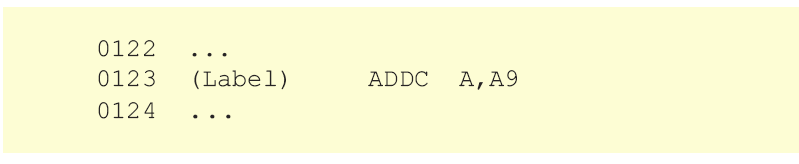
Before execution: A= C3h (195 dec.) C=1
After execution: A= 6Dh (109 dec.) AC=0, C=1, OV=1
After execution: A= 6Dh (109 dec.) AC=0, C=1, OV=1
AJMP addr11 - Absoulte jump
addr11: Jump address
Description: Program continues execution after executing a jump to the specified address. Similar to the ACALL instruction, the jump must be executed within the same 2K byte block of program memory starting from the first byte of the instruction following AJMP.
Syntax: AJMP address (label);
Bytes: 2 (instruction code, jump address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
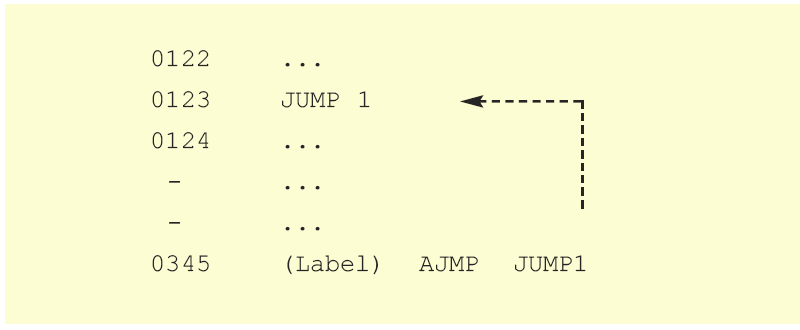
Before execution: PC=0345h SP=07h
After execution: PC=0123h SP=09h
After execution: PC=0123h SP=09h
ANL A,Rn - AND register to the accumulator
A: accumulator
Rn: any R register (R0-R7)
Rn: any R register (R0-R7)
Description: Instruction performs logic AND operation between the accumulator and Rn register. The result is stored in the accumulator.
Syntax: ANL A,Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
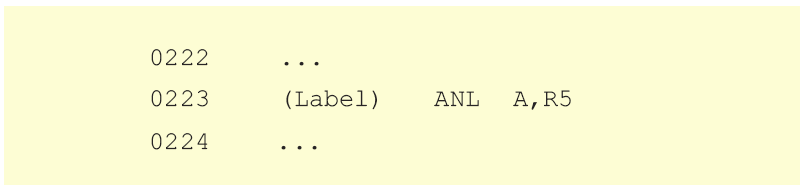
Before execution: A= C3h (11000011 Bin.)
R5= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
R5= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
ANL A,direct - AND direct byte to the accumulator
A: accumulator
Direct: arbitrary register with address 0 - 255 (0 - FFh)
Direct: arbitrary register with address 0 - 255 (0 - FFh)
Description: Instruction performs logic AND operation between the accumulator and drect register. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (o-127 dec.). The result is stored in the accumulator.
Syntax: ANL A,direct;
Byte: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
Byte: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
EXAMPLE:
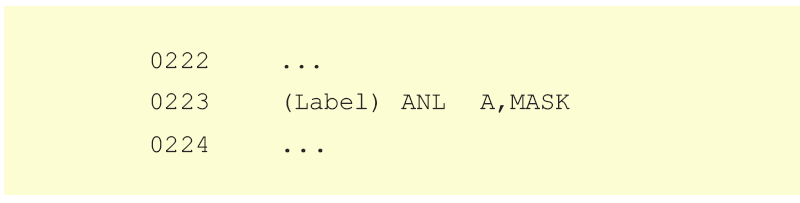
Before execution: A= C3h (11000011 Bin.)
MASK= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
MASK= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
ANL A,@Ri - AND indirect RAM to the accumulator
A: accumulator
Ri: Register R0 or R1
Ri: Register R0 or R1
Description: Instruction performs logic AND operation between the accumulator and register. As it is indirect addressing, the register address is stored in the Ri register (R0 or R1). The result is stored in the accumulator.
Syntax: ANL A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
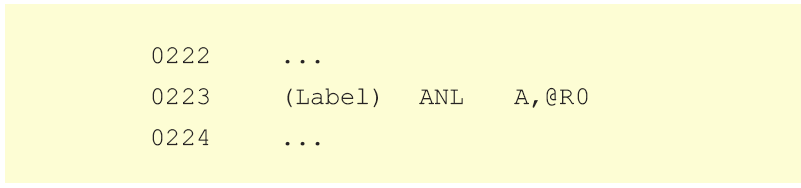
Register address SUM = 4Fh R0=4Fh
Before execution: A= C3h (11000011 Bin.)
R0= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
Before execution: A= C3h (11000011 Bin.)
R0= 55h (01010101 Bin.)
After execution: A= 41h (01000001 Bin.)
ANL A,#data - AND immediate data to the accumulator
A: accumulator
Data: constant in the range of 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Description: Instruction performs logic AND operation between the accumulator and data. The result is stored in the accumulator.
Syntax: ANL A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
EXAMPLE:
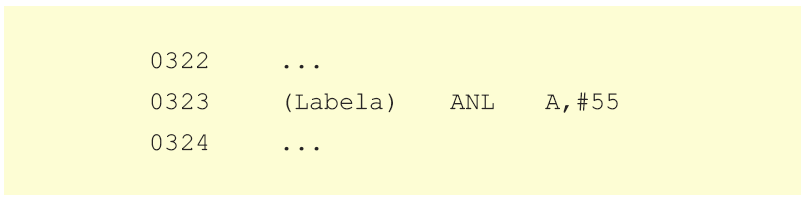
Before execution: A= C3h (11000011 Bin.)
After execution: A= 41h (01000001 Bin.)
After execution: A= 41h (01000001 Bin.)
ANL direct,A - AND accumulator to direct byte
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs logic AND operation between direct byte and accumulator. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the direct byte.
Syntax: ANL register address,A;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected.
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected.
EXAMPLE:
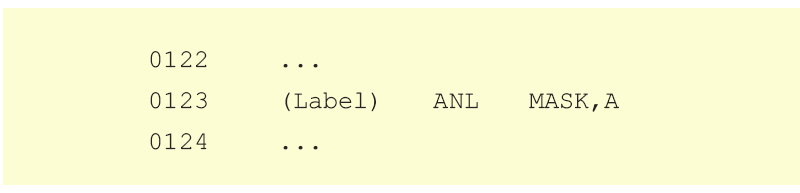
Before execution: A= C3h (11000011 Bin.)
MASK= 55h (01010101 Bin.)
After execution: MASK= 41h (01000001 Bin.)
MASK= 55h (01010101 Bin.)
After execution: MASK= 41h (01000001 Bin.)
ANL direct,#data - AND immediate data to direct byte
Direct: Arbitrary register with address 0 - 255 (0 - FFh)
Data: constant in the range between 0-255 (0-FFh)
Data: constant in the range between 0-255 (0-FFh)
Description: Instruction performs logic AND operation between direct byte and data. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the direct byte.
Syntax: ANL register address ,#data;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags are affected;
EXAMPLE:
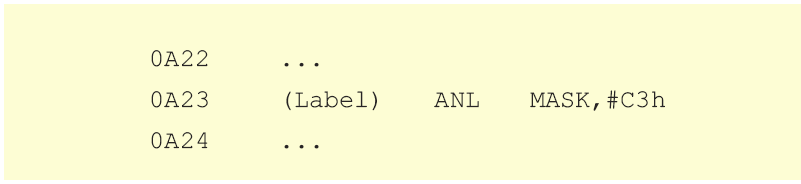
Before execution: X= C3h (11000011 Bin.) MASK= 55h (01010101 Bin.) After execution: MASK= 41h (01000001 Bin.)
ANL C,bit - AND direct bit to the carry flag
C: Carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction performs logic AND operation between the direct bit and the carry flag.
BIT | C | C AND BIT |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Syntax: ANL C, bit address;
Bytes: 2 (instruction code, bit address);
STATUS register flags: C;
Bytes: 2 (instruction code, bit address);
STATUS register flags: C;
EXAMPLE:
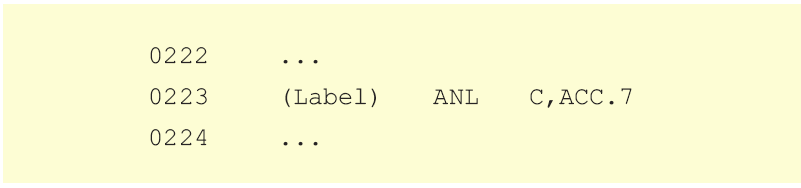
Before execution: ACC= 43h (01000011 Bin.)
C=1
After execution: ACC= 43h (01000011 Bin.)
C=0
C=1
After execution: ACC= 43h (01000011 Bin.)
C=0
ANL C,/bit - AND complements of direct bit to the carry flag
C: carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction performs logic AND operation between inverted addressed bit and the carry flag. The result is stored in the carry flag.
BIT | BIT | C | C AND BIT |
---|---|---|---|
0 | 1 | 0 | 0 |
0 | 1 | 1 | 1 |
1 | 0 | 0 | 0 |
1 | 0 | 1 | 0 |
Syntax: ANL C,/[bit address];
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
EXAMPLE:
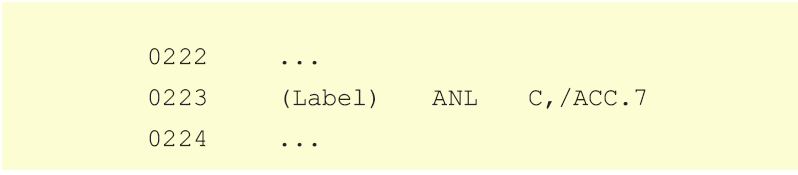
Before execution: ACC= 43h (01000011 Bin.)
C=1
After execution: ACC= 43h (01000011 Bin.)
C=1
C=1
After execution: ACC= 43h (01000011 Bin.)
C=1
CJNE A,direct,rel - Compares direct byte to the accumulator and jumps if not equal
A: accumulator
Direct: arbitrary register with address 0-255 (0-FFh)
addr: jump address
Direct: arbitrary register with address 0-255 (0-FFh)
addr: jump address
Description: Instruction first compares the number in the accumulator with the directly addressed byte. If they are equal, the program proceeds with execution. Otherwise, a jump to the specified address will be executed. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to +127 locations relative to the first following instruction).
Syntax: CJNE A,direct,[jump address];
Bytes: 3 (instruction code, direct byte address, jump address);
STATUS register flags: C;
Bytes: 3 (instruction code, direct byte address, jump address);
STATUS register flags: C;
EXAMPLE:
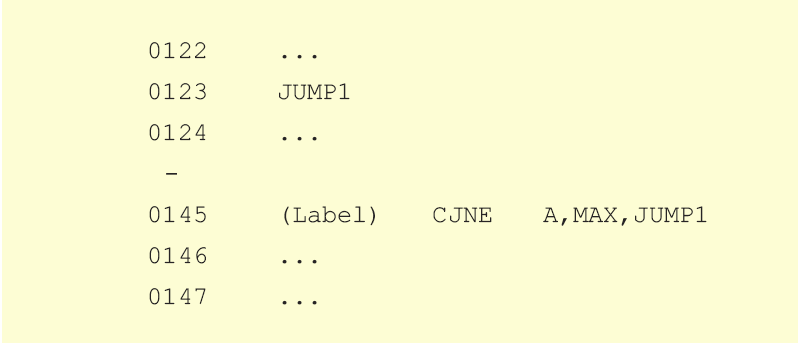
Before execution: PC=0145h A=27h
After execution: if MAX≠27: PC=0123h
If MAX=27: PC=0146h
After execution: if MAX≠27: PC=0123h
If MAX=27: PC=0146h
CJNE A,#data,rel - Compares immediate data to the accumulator and jumps if not equal
A: accumulator
Data: constant in the range of 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Description: Instruction first compares the number in the accumulator with the immediate data. If they are equal, the program proceeds with execution. Otherwise, a jump to the specified address will be executed. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to +127 locations relative to the first following instruction).
Syntax: CJNE A,X,[jump address];
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
EXAMPLE:
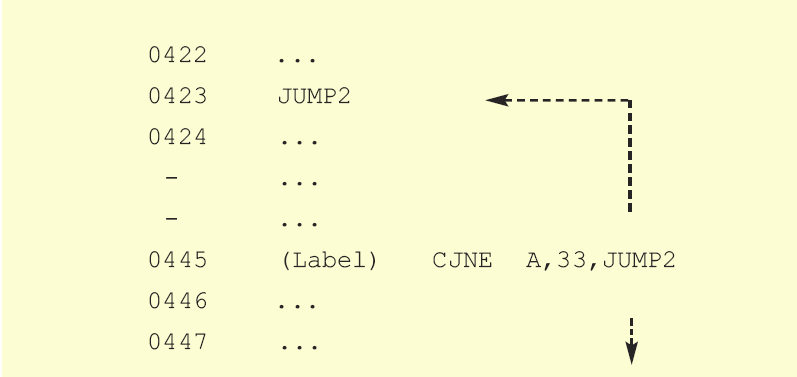
Before execution: PC=0445h
After execution: If A≠33: PC=0423h
If A=33: PC=0446h
After execution: If A≠33: PC=0423h
If A=33: PC=0446h
CJNE Rn,#data,rel - Compares immediate data to the register Rn and jumps if not equal
Rn: Any R register (R0-R7)
Data: Constant in the range of 0 - 255 (0-FFh)
addr: Jump address
Data: Constant in the range of 0 - 255 (0-FFh)
addr: Jump address
Description: Instruction first compares immediate data to the register Rn. If they are equal, the program proceeds with execution. Otherwise, a jump to the specified address will be executed. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to + 127 locations relative to the first following instruction).
Syntax: CJNE Rn,data,[jump address];
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
EXAMPLE:
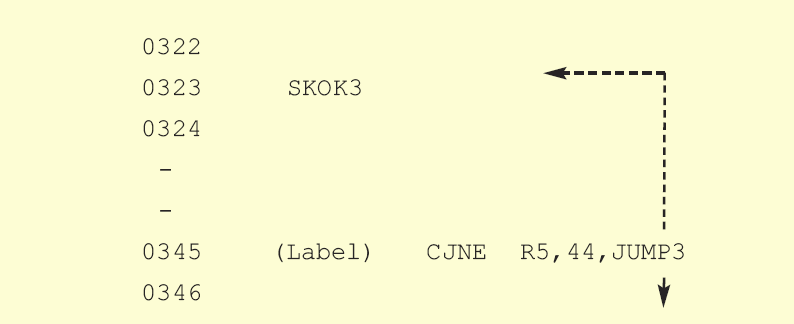
Before execution: PC=0345h
After execution: If R5≠44h: PC=0323h
If R5=44h: PC=0346h
After execution: If R5≠44h: PC=0323h
If R5=44h: PC=0346h
CJNE @Ri,#data,rel - Compares immediate data to indirectly addressed register and jumps if not equal
Ri: Register R0 or R1
Data: Constant in the range of 0 - 255 (0-FFh)
Data: Constant in the range of 0 - 255 (0-FFh)
Description: This instruction first compares immediate data to indirectly addressed register. If they are equal, the program proceeds with execution. Otherwise, a jump to the specified address in the program will be executed. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to +127 locations relative to the next instruction).
Syntax: CJNE @Ri,data,[jump address];
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
Bytes: 3 (instruction code, data, jump address);
STATUS register flags: C;
EXAMPLE:
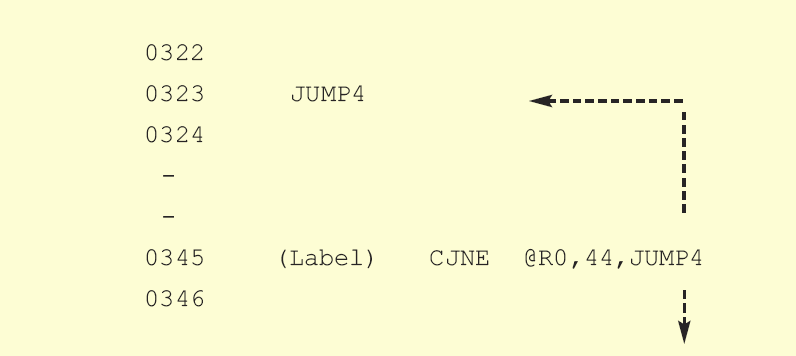
Before execution: Register Address SUM=F3h
PC=0345h R0=F3h
After execution: If SUM≠44h: PC=0323h
If SUM=44h: PC=0346h
PC=0345h R0=F3h
After execution: If SUM≠44h: PC=0323h
If SUM=44h: PC=0346h
CLR A - Clears the accumulator
A: accumulator
Description: Instruction clears the accumulator.
Syntax: CLR A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected.
Byte: 1 (instruction code);
STATUS register flags: No flags are affected.
EXAMPLE:
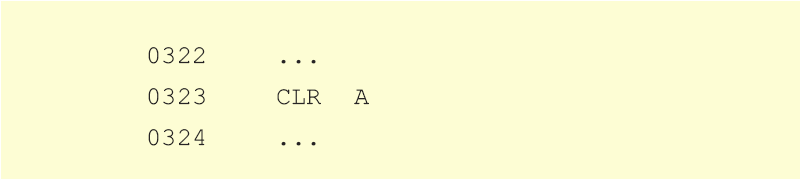
After execution: A=0
CLR C - clears the carry flag
C: Carry flag
Description: Instruction clears the carry flag.
Syntax: CLR C;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:
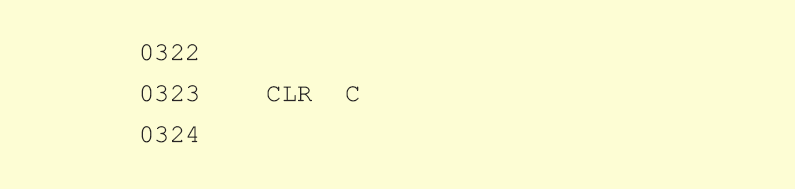
After execution: C=0
CLR bit - clears the direct bit
Bit: any bit of RAM
Description: Instruction clears the specified bit.
Syntax: CLR [bit address];
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected.
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected.
EXAMPLE:
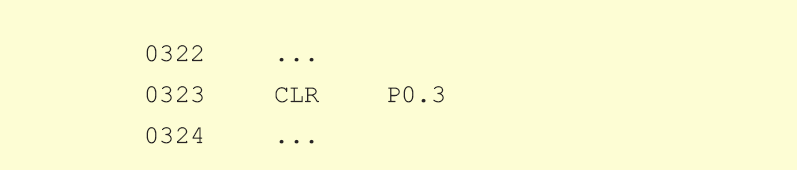
Before execution: P0.3=1 (input pin)
After execution: P0.3=0 (output pin)
After execution: P0.3=0 (output pin)
CPL A - Complements the accumulator
A: accumulator
Description: Instruction complements all the bits in the accumulator (1==>0, 0==>1).
Syntax: CPL A;
Bytes: 1 (instruction code);
STATUS register flags: No flags are affected.
Bytes: 1 (instruction code);
STATUS register flags: No flags are affected.
EXAMPLE:
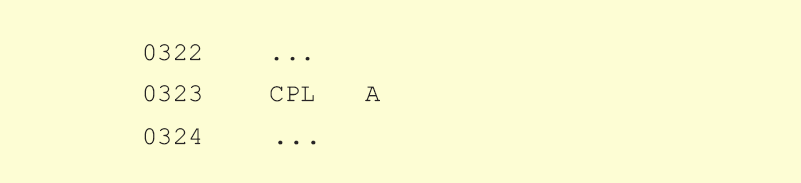
Before execution: A= (00110110)
After execution: A= (11001001)
After execution: A= (11001001)
CPL bit - Complements the direct bit
Bit: any bit of RAM
Description: Instruction coplements the specified bit of RAM (0==>1, 1==>0).
Syntax: CPL [bit address];
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
EXAMPLE:
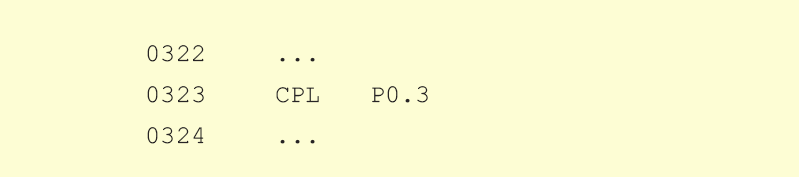
Before execution: P0.3=1 (input pin)
After execution: P0.3=0 (output pin)
After execution: P0.3=0 (output pin)
CPL C - Complements the carry flag
C: Carry flag
Description: Instruction complements the carry flag (0==>1, 1==>0).
Syntax: CPL C;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:
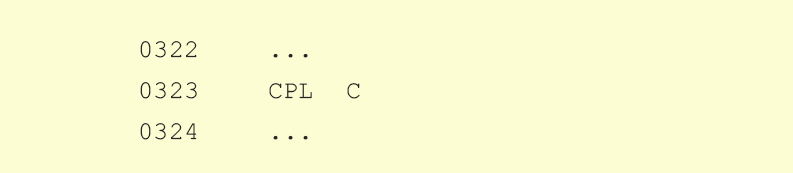
Before execution: C=1
After execution: C=0
After execution: C=0
DA A - Decimal adjust accumulator
A: accumulator
Description: Instruction adjusts the contents of the accumulator to correspond to a BCD number after two BCD numbers have been added by the ADD and ADDC instructions. The result in form of two 4-digit BCD numbers is stored in the accumulator.
Syntax: DA A;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:
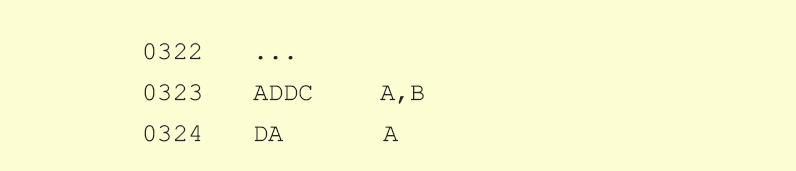
Before execution: A=56h (01010110) 56 BCD
B=67h (01100111) 67BCD
After execution: A=BDh (10111101)
After BCD conversion: A=23h (00100011), C=1 (Overflow)
(C+23=123) = 56+67
B=67h (01100111) 67BCD
After execution: A=BDh (10111101)
After BCD conversion: A=23h (00100011), C=1 (Overflow)
(C+23=123) = 56+67
DEC A - Decrements the accumulator by 1
A: accumulator
Description: Instruction decrements the value in the accumulator by 1. If there is a 0 in the accumulator, the result of the operation is FFh. (255 dec.)
Syntax: DEC A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
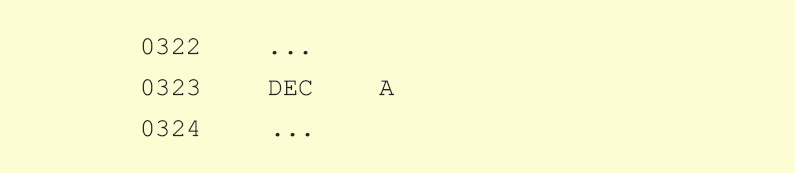
Before execution: A=E4h
After execution: A=E3h
After execution: A=E3h
DEC Rn - Decrements the register Rn by 1
Rn: any R register (R0-R7)
Description: Instruction decrements the value in the Rn register by 1. If there is a 0 in the register, the result of the operation will be FFh. (255 dec.)
Syntax: DEC Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
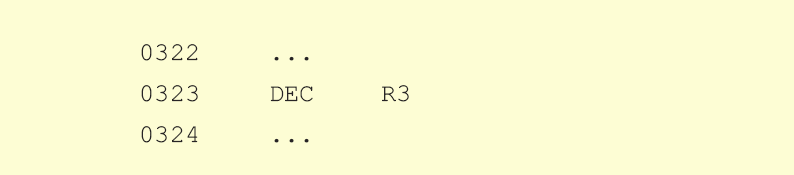
Before execution: R3=B0h
After execution: R3=AFh
After execution: R3=AFh
DEC direct - Decrements the direct byte by 1
Direct: arbitrary register with address 0-255 (0-FFh)
Description: Instruction decrements the value of directly addressed register by 1. As it is direct addressing, the register must be within the first 255 locations of RAM. If there is a 0 in the register, the result will be FFh.
Syntax: DEC [register address];
Byte: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
Byte: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
EXAMPLE:
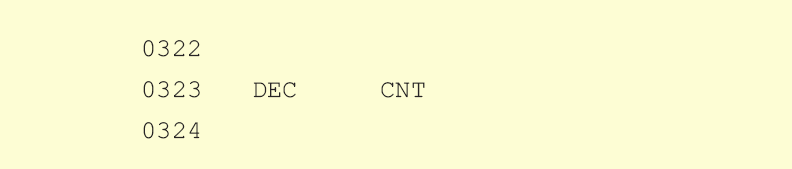
Before execution: CNT=0
After execution: CNT=FFh
After execution: CNT=FFh
DIV AB - Divides the accumulator by the register B
A: accumulator
B: Register B
B: Register B
Description: Instruction divides the value in the accumulator by the value in the B register. After division the integer part of result is stored in the accumulator while the register contains the remainder. In case of dividing by 1, the flag OV is set and the result of division is unpredictable. The 8-bit quotient is stored in the accumulator and the 8-bit remainder is stored in the B register.
Syntax: DIV AB;
Byte: 1 (instruction code);
STATUS register flags: C, OV;
Byte: 1 (instruction code);
STATUS register flags: C, OV;
EXAMPLE:
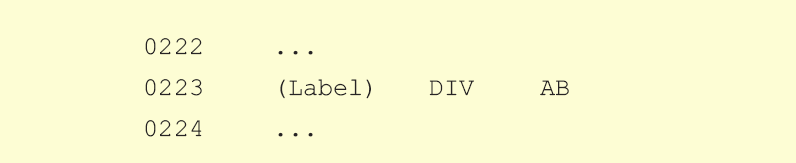
Before execution: A=FBh (251dec.) B=12h (18 dec.)
After execution: A=0Dh (13dec.) B=11h (17dec.)
13·18 + 17 =251
After execution: A=0Dh (13dec.) B=11h (17dec.)
13·18 + 17 =251
DEC @Ri - Decrements the indirect RAM by 1
Ri: Register R0 or R1
Description: This instruction decrements the value in the indirectly addressed register of RAM by 1. The register address is stored in the Ri register (R0 or R1). If there is a 0 in the register, the result will be FFh.
Syntax: DEC @Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
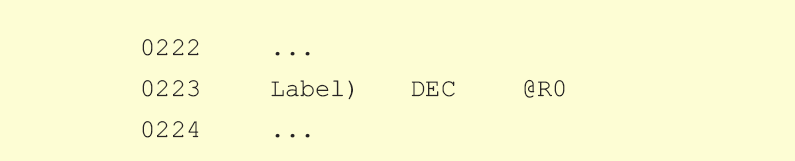
Register Address CNT = 4Fh R0=4Fh
Before execution: CNT=35h
After execution: CNT= 34h
Before execution: CNT=35h
After execution: CNT= 34h
DJNZ direct,rel - Decrements direct byte by 1 and jumps if not 0
Direct: arbitrary register with address 0-255 (0-FFh)
addr: Jump address
addr: Jump address
Description: This instruction first decrements value in the register. If the result is 0, the program proceeds with execution. Otherwise, a jump to the specified address in the program will be executed. As it is direct addressing, the register must be within the first 255 locations of RAM. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to +127 locations relative to the first following instruction).
Syntax: DJNZ direct,[jump address];
Bytes: 3 (instruction code, direct, jump address);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, direct, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
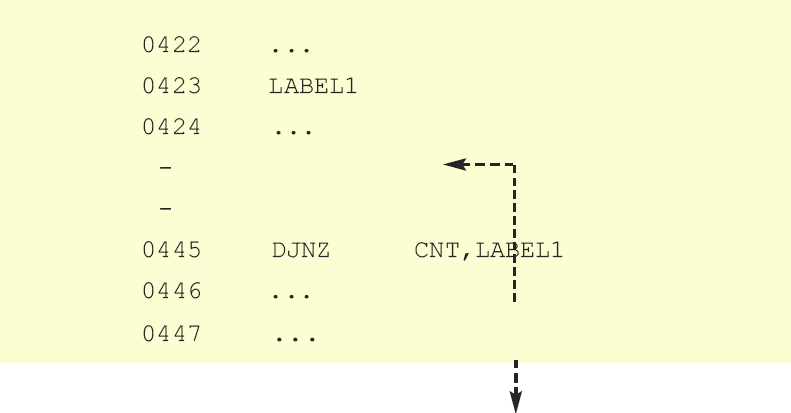
Before execution: PC=0445h
After execution: If CNT≠0: PC=0423h
If CNT=0: PC=0446h
After execution: If CNT≠0: PC=0423h
If CNT=0: PC=0446h
DJNZ Rn,rel - Decrements the Rn register by 1 and jumps if not 0
Rn: any R register (R0-R7)
addr: jump address
addr: jump address
Description: This instruction first decrements the value in the Rn register. If the result is 0, the program proceeds with execution. Otherwise, a jump to the specified address in the program will be executed. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (- 128 to +127 locations relative to the first following instruction).
Syntax: DJNZ Rn, [jump address];
Bytes: 2 (instruction code, jump address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
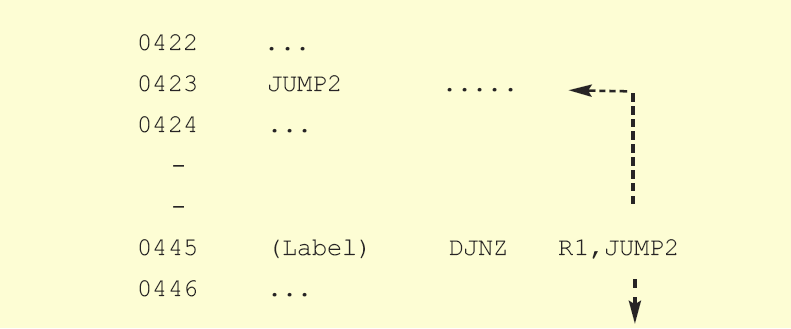
Before execution: PC=0445h
After execution: If R1≠0: PC=0423h
If R1=0: PC=0446h
After execution: If R1≠0: PC=0423h
If R1=0: PC=0446h
INC Rn - Increments the Rn register by 1
Rn: any R register (R0-R7)
Description: Instruction increments the value in the Rn register by 1. If the register includes the number 255, the result of the operation will be 0.
Syntax: INC Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
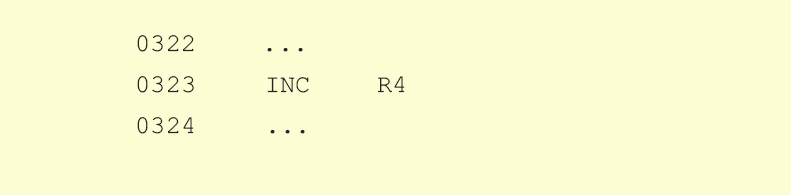
Before execution: R4=18h
After execution: R4=19h
After execution: R4=19h
INC A - Increments the accumulator by 1
A: accumulator
Description: This instruction increments the value in the accumulator by 1. If the accumulator includes the number 255, the result of the operation will be 0.
Syntax: INC A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
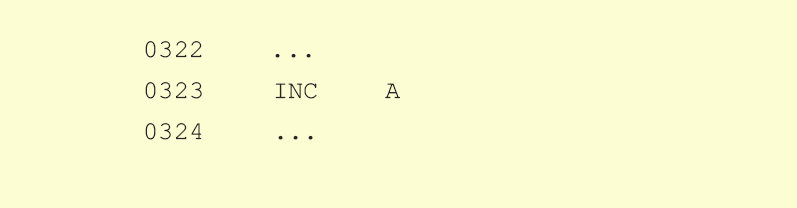
Before execution: A=E4h
After execution: A=E5h
After execution: A=E5h
INC @Ri - Increments the value of indirectly addressed register of RAM by 1
Ri: Register R0 or R1
Description: This instruction increments the value in the directly addressed register of RAM by 1. The register address is stored in the Ri Register (R0 or R1). If the register includes the number 255, the result of the operation will be 0.
Syntax: INC @Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
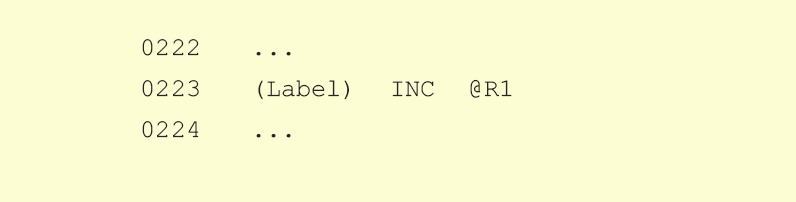
Register Address CNT = 4Fh
Before execution: CNT=35h R1=4Fh
After execution: CNT=36h
Before execution: CNT=35h R1=4Fh
After execution: CNT=36h
INC direct - Increments the direct byte by 1
Direct: arbitrary register with address 0-255 (0-FFh)
Description: Instruction increments the direct byte by 1. If the register includes the number 255, the result of the operation will be 0. As it is direct addressing, the register must be within the first 255 RAM locations.
Syntax: INC direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
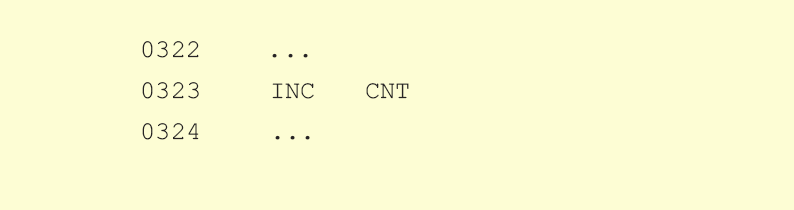
Before execution: CNT=33h
After execution: CNT=34h
After execution: CNT=34h
JB bit,rel - Jump if direct bit is set
addr: Jump address
Bit: any bit of RAM
Bit: any bit of RAM
Description: If the bit is set, a jump to the specified address will be executed. Otherwise, if the value of bit is 0, the program proceeds with the next instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-128 to + 127 locations relative to the first following instruction).
Syntax: JB bit, [jump address];
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
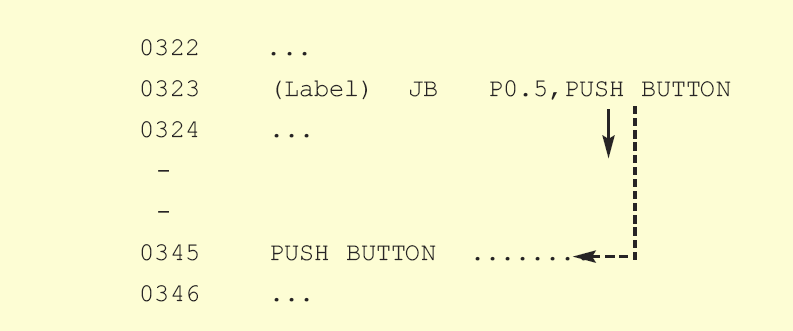
Before execution: PC=0323h
After execution: If P0.5=0: PC=0324h
If P0.5=1: PC=0345h
After execution: If P0.5=0: PC=0324h
If P0.5=1: PC=0345h
INC DPTR - Increments the Data Pointer by 1
DPTR: Data Pointer
Description: Instruction increments the value of the 16-bit data pointer by 1. This is the only 16-bit register upon which this operation can be performed.
Syntax: INC DPTR;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
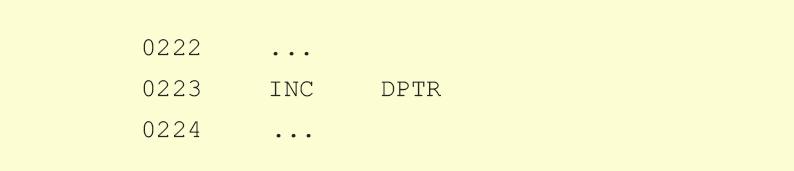
Before execution: DPTR = 13FF (DPH = 13h DPL = FFh )
After execution: DPTR = 1400 (DPH = 14h DPL = 0)
After execution: DPTR = 1400 (DPH = 14h DPL = 0)
JC rel - Jump if carry flag is set
addr: Jump address
Description: Instruction first checks if the carry flag is set. If set, a jump to the specified address is executed. Otherwise, the program proceeds with the next instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JC [jump address];
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
EXAMPLE:
![JC [jump address] JC [jump address]](http://www.mikroe.com/img/publication/8051-books/programming-8051-mcu/chapter/ch3/43.gif)
Before instruction: PC=0323h
After instruction: If C=0: PC=0324h
If C=1: PC=0345h
After instruction: If C=0: PC=0324h
If C=1: PC=0345h
JBC bit,rel - Jump if direct bit is set
Bit: any bit of RAM
addr: Jump Address
addr: Jump Address
Description: This instruction first checks if the bit is set. If set, a jump to the specified address is executed and the bit is cleared. Otherwise, the program proceeds with the first following instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JBC bit, [jump address];
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
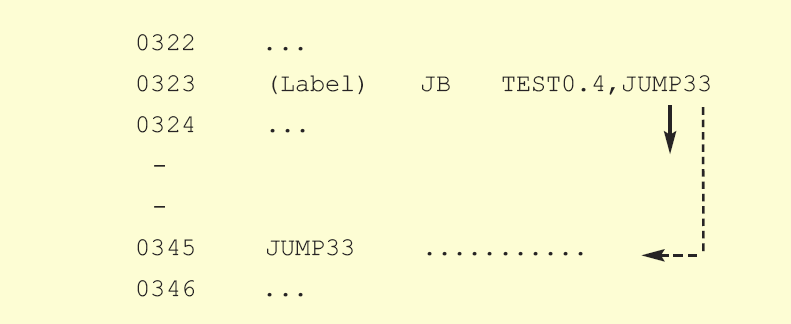
Before execution: PC=0323h
After execution: If TEST0.4=1: PC=0345h, TEST0.4=0
If TEST0.4=0: PC=0324h, TEST0,4=0
After execution: If TEST0.4=1: PC=0345h, TEST0.4=0
If TEST0.4=0: PC=0324h, TEST0,4=0
JNB bit,rel - Jump if direct bit is not set
addr: Jump address
Bit: any bit of RAM
Bit: any bit of RAM
Description: If the bit is cleared, a jump to the specified address will be executed. Otherwise, if the bit value is 1, the program proceeds with the first following instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JNB bit,[jump address];
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, bit address, jump address);
STATUS register flags: No flags are affected;
EXAMPLE:
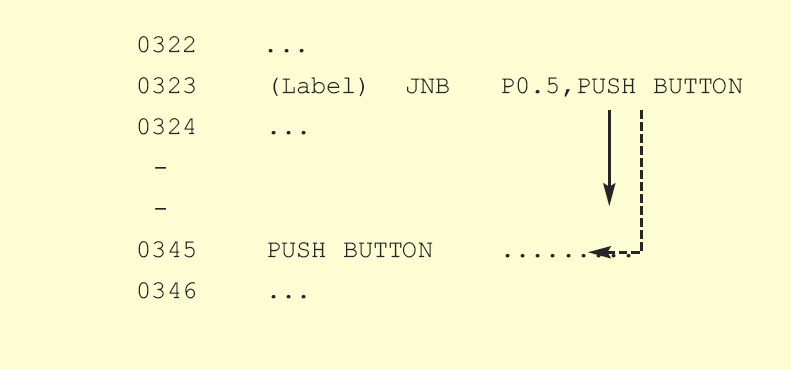
Before execution: PC=0323h
After execution: If P0.5=1: PC=0324h
If P0.5=0: PC=0345h
After execution: If P0.5=1: PC=0324h
If P0.5=0: PC=0345h
JMP @A+DPTR - Jump indirect relative to the DPTR
A: accumulator
DPTR: Data Pointer
DPTR: Data Pointer
Description: This instruction causes a jump to the address calculated by adding value stored in the accumulator to the 16-bit number in the DPTR Register. It is used with complex program branching where the accumulator affects jump address, for example when reading a table. Neither accumulator nor DPTR register are affected.
Syntax: JMP @A+DPTR;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
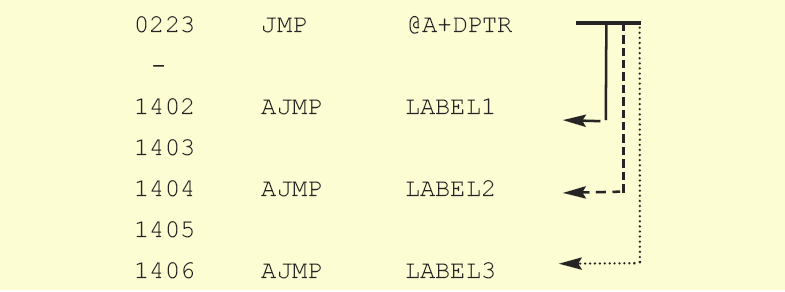
Before execution: PC=223 DPTR=1400h
After execution: PC = 1402h if A=2
PC = 1404h if A=4
PC = 1406h if A=6
After execution: PC = 1402h if A=2
PC = 1404h if A=4
PC = 1406h if A=6
Note:
As instructions AJMP LABELS occupy two locations each, the values in the accumulator specifying them must be different from each other by 2.
As instructions AJMP LABELS occupy two locations each, the values in the accumulator specifying them must be different from each other by 2.
JNZ rel - Jump if accumulator is not zero
addr: Jump Address
Description: This instruction checks if the value stored in the accumulator is 0. If not, a jump to the specified address will be executed. Otherwise, the program proceeds with the first following instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JNZ [jump address]:
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
EXAMPLE:
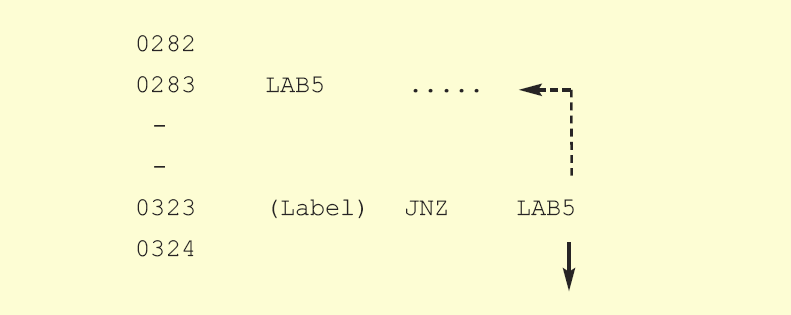
Before execution: PC=0323h
After execution: If A=0: PC=324h
If A≠0: PC=283h
After execution: If A=0: PC=324h
If A≠0: PC=283h
JNC rel - Jump if carry flag is not set
addr: Jump Address
Description: This instruction first checks whether the carry flag is set. If not, a jump to the specified address will be executed. Otherwise, the program proceeds with the first following instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JNC [jump address];
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
EXAMPLE:
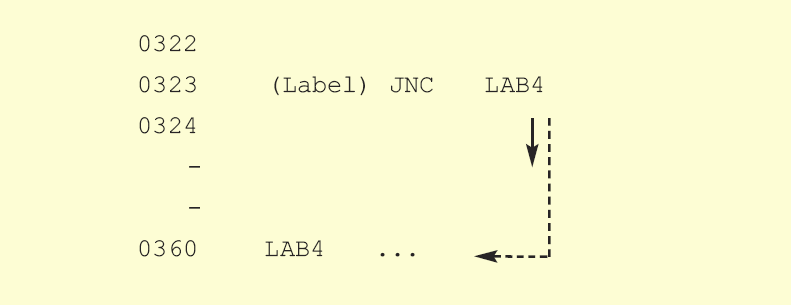
Before execution: PC=0323h
After execution: If C=0: PC=360h
If C=1: PC=324h
After execution: If C=0: PC=360h
If C=1: PC=324h
LCALL addr16 - Long subroutine call
addr16: Subroutine Address
Description: This instruction unconditionally calls a subroutine located at the specified address. The current address and the start of the subroutine called can be located anywhere within the memory space of 64K.
Syntax: LCALL [subroutine name];
Bytes: 3 (instruction code, address (15-8), address (7-0));
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, address (15-8), address (7-0));
STATUS register flags: No flags are affected;
EXAMPLE:
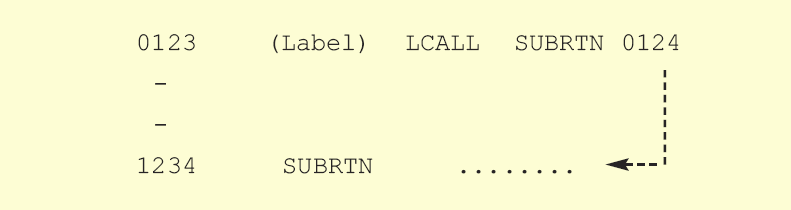
Before execution: PC=0123h
After execution: PC=1234h
After execution: PC=1234h
JZ rel - Jump if accumulator is zero
addr: Jump Address
Description: The instruction checks whether the value stored in the accumulator is 0. If yes, a jump to the specified address will be executed. Otherwise, the program proceeds with the following instruction. This is a short jump instruction, which means that the address of a new location must be relatively near the current one (-129 to + 127 locations relative to the first following instruction).
Syntax: JZ [jump address];
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
EXAMPLE:
![JZ [jump address] JZ [jump address]](http://www.mikroe.com/img/publication/8051-books/programming-8051-mcu/chapter/ch3/50.gif)
Before execution: PC=0323h
After execution: If A0: PC=324h
If A=0: PC=283h
After execution: If A0: PC=324h
If A=0: PC=283h
MOV A,Rn - Moves the Rn register to the accumulator
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Description: The instruction moves the Rn register to the accumulator. The Rn register is not affected.
Syntax: MOV A,Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:

Before execution: R3=58h
After execution: R3=58h A=58h
After execution: R3=58h A=58h
LJMP addr16 - Long jump
addr16: jump address
Description: Instruction causes a jump to the specified 16-bit address.
Syntax: LJMP [jump address];
Bytes: 3 (instruction code, address (15-8), address (7-0));
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, address (15-8), address (7-0));
STATUS register flags: No flags are affected;
EXAMPLE:
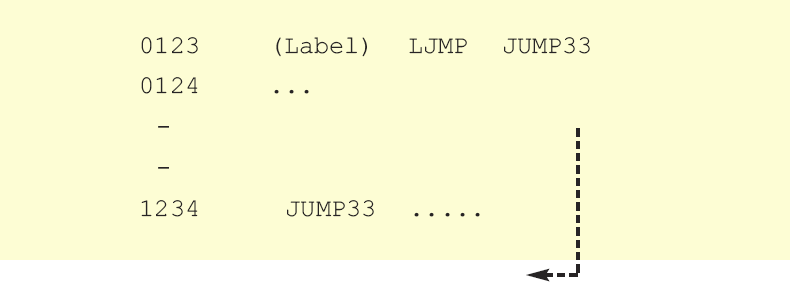
Before execution: PC=0123h
After execution: PC=1234h
After execution: PC=1234h
MOV A,@Ri - Moves the indirect RAM to the accumulator
Ri: Register R0 or R1
A: accumulator
A: accumulator
Description: Instruction moves the indirectly addressed register of RAM to the accumulator. The register address is stored in the Ri register (R0 or R1). The result is stored in the accumulator. The register is not affected.
Syntax: MOV A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
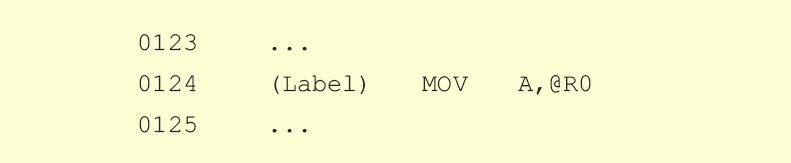
Register Address SUM=F2h R0=F2h
Before execution: SUM=58h
After execution: A=58h SUM=58h
Before execution: SUM=58h
After execution: A=58h SUM=58h
MOV A,direct - Moves the direct byte to the accumulator
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction moves the direct byte to the accumulator. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.). After executing the instruction, the register is not affected.
Syntax: MOV A,direct;
Byte: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Byte: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
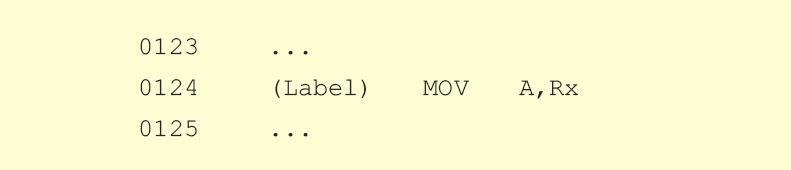
Before execution: Rx=68h
After execution: Rx=68h A=68h
After execution: Rx=68h A=68h
MOV Rn,A - Moves the accumulator to the Rn register
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Desription: Instruction moves the accumulator to the Rn register. The accumulator is not affected.
Syntax: MOV Rn,A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
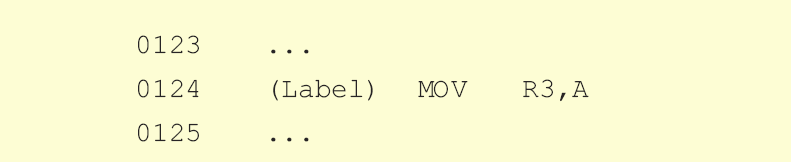
Before execution: A=58h
After execution: R3=58h A=58h
After execution: R3=58h A=58h
MOV A,#data - Moves the immediate data to the accumulator
A: accumulator
Data: Constant in the range of 0-255 (0-FFh)
Data: Constant in the range of 0-255 (0-FFh)
Desription: Instruction moves the immediate data to the accumulator.
Syntax: MOV A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
EXAMPLE:
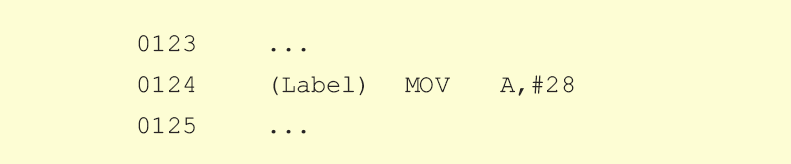
After execution: A=28h
MOV Rn,#data - Moves the immediate data to the Rn register
Rn: any R register (R0-R7) Data: Constant in the range of 0-255 (0-FFh)
Description: Instruction moves the immediate data to the Rn register.
Syntax: MOV Rn,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
EXAMPLE:
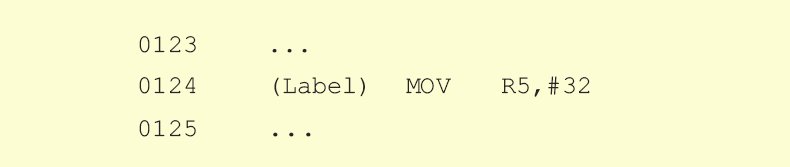
After execution: R5=32h
MOV Rn,direct - Moves the direct byte to the Rn register
Rn: Any R registar (R0-R7)
Direct: arbitrary register with address 0-255 (0-FFh)
Direct: arbitrary register with address 0-255 (0-FFh)
Description: Instruction moves the direct byte to the Rn register. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.). After executing the instruction, the register is not affected.
Syntax: MOV Rn,direct;
Bytes: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct);
STATUS register flags: No flags are affected;
EXAMPLE:
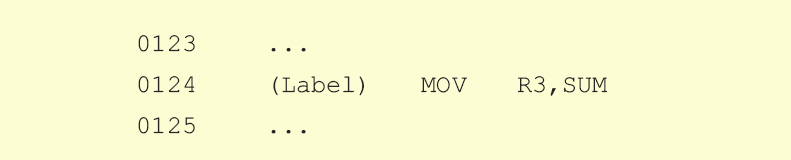
Before execution: SUM=58h
After execution: SUM=58h R3=58h
After execution: SUM=58h R3=58h
MOV direct,Rn - Moves the Rn register to the direct byte
Rn: any R register (R0-R7)
Direct: arbitrary register with address 0-255 (0 - FFh)
Direct: arbitrary register with address 0-255 (0 - FFh)
Description: Instruction moves the Rn register to the direct byte. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.). After executing the instruction, the register is not affected.
Syntax: MOV direct,Rn;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
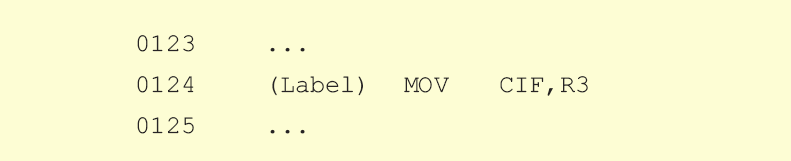
Before execution: R3=18h
After execution: R3=18h CIF=18h
After execution: R3=18h CIF=18h
MOV direct,A - Moves the accumulator to the direct byte
Direct: arbitrary register with address 0-255 (0 - FFh)
A: accumulator
A: accumulator
Description: Instruction moves the accumulator to the direct byte. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.). After executing the instruction, the register is not affected.
Syntax: MOV direct,A;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
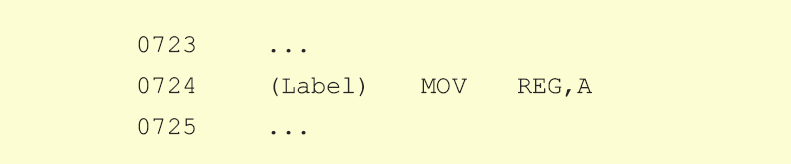
Before execution: A=98h
After execution: A=98h REG=98h
After execution: A=98h REG=98h
MOV direct,@Ri - Moves the indirect RAM to the direct byte
Direct: arbitrary register with address 0-255 (0 - FFh)
Ri: Register R0 or R1
Ri: Register R0 or R1
Description: Instruction moves the indirectly adressed register of RAM to the direct byte. The register is not affected.
Syntax: MOV direct,@Ri;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
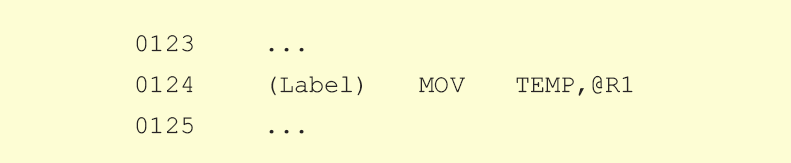
Register Address SUM=F3
Before execution: SUM=58h R1=F3
After execution: SUM=58h TEMP=58h
Before execution: SUM=58h R1=F3
After execution: SUM=58h TEMP=58h
MOV direct1,direct2 - Moves the direct byte to the direct byte
Direct: Arbitrary register with address 0-255 (0-FFh)
Direct: Arbitrary register with address 0-255 (0-FFh)
Direct: Arbitrary register with address 0-255 (0-FFh)
Description: Instruction moves the direct byte to another direct byte. As it is direct addressing, both registers can be any SFRs or general-purpose registers with address 0-7Fh. (0-127 dec.). The direct1 is not affected.
Syntax: MOV direct1,direct2;
Bytes: 3 (instruction code, direct1 address, direct2 address);
STATUS register flags: No flags are affected.
Bytes: 3 (instruction code, direct1 address, direct2 address);
STATUS register flags: No flags are affected.
EXAMPLE:
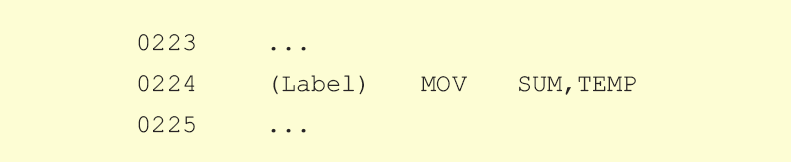
Before execution: TEMP=58h
After execution: TEMP=58h SUM=58h
After execution: TEMP=58h SUM=58h
MOV @Ri,A - Moves the accumulator to the indirect RAM
A: accumulator
Ri: register R0 or R1
Ri: register R0 or R1
Description: Instruction moves the accumulator to the indirectly addressed register of RAM. The register address is stored in the Ri register (R0 or R1). After executing the instruction, the accumulator is not affected.
Syntax: MOV @Ri,A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
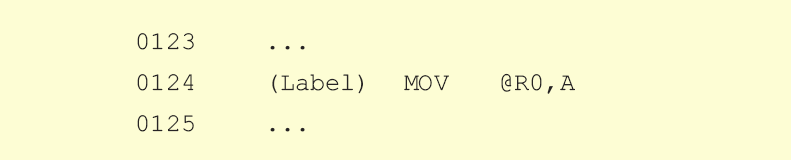
Register Address SUM=F2h
Before execution: R0=F2h A=58h
After execution: SUM=58h A=58h
Before execution: R0=F2h A=58h
After execution: SUM=58h A=58h
MOV direct,#data - Moves the immediate data to the direct byte
Direct: Arbitrary register with address 0-255 (0-FFh)
Data: Constant in the range of 0-255 (0-FFh)
Data: Constant in the range of 0-255 (0-FFh)
Description: Instruction moves the immediate data to the direct byte. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.).
Syntax: MOV direct,#data;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags are affected;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags are affected;
EXAMPLE:

After execution: TEMP=22h
MOV @Ri,#data - Moves the immediate data to the indirect RAM
Ri: Register R0 or R1
Data: Constant in the range of 0-255 (0-FFh)
Data: Constant in the range of 0-255 (0-FFh)
Description: Instruction moves the immediate data to the idirectly addressed register of RAM. The register address is stored in the Ri register (R0 or R1).
Syntax: MOV @Ri,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
EXAMPLE:

Register address TEMP=E2h
Before execution: R1=E2h
After execution: TEMP=44h
Before execution: R1=E2h
After execution: TEMP=44h
MOV @Ri,direct - Moves the direct byte to the indirect RAM
Direct: Arbitrary register with address 0-255 (0-FFh)
Ri: Register R0 or R1
Ri: Register R0 or R1
Description: Instruction moves the direct byte to a register the address of which is stored in the Ri register (R0 or R1). After executing the instruction, the direct byte is not affected.
Syntax: MOV @Ri,direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:

Register address TEMP=E2h
Before execution: SUM=58h R1=E2h
After execution: SUM=58h TEMP=58h
Before execution: SUM=58h R1=E2h
After execution: SUM=58h TEMP=58h
MOV bit,C - Moves the carry flag to the direct bit
C: Carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction moves the carry flag to the direct bit. After executing the instruction, the carry flag is not affected.
Syntax: MOV bit,C;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags are affected;
EXAMPLE:
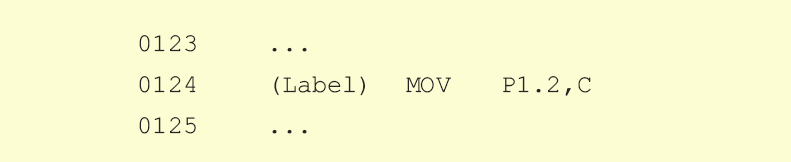
After execution: If C=0 P1.2=0
If C=1 P1.2=1
If C=1 P1.2=1
MOV C,bit - Moves the direct bit to the carry flag
C: Carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction moves the direct bit to the carry flag. After executing the instruction, the bit is not affected.
Syntax: MOV C,bit;
Bytes: 2 (instruction code, bit address);
STATUS register flags: C;
Bytes: 2 (instruction code, bit address);
STATUS register flags: C;
EXAMPLE:
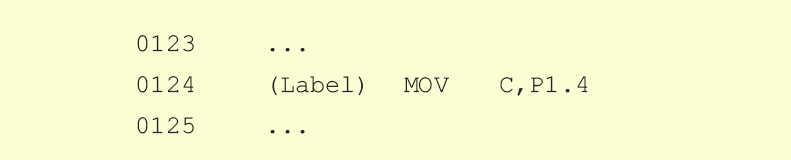
After execution: If P1.4=0 C=0
If P1.4=1 C=1
If P1.4=1 C=1
MOVC A,@A+DPTR - Moves the code byte relative to the DPTR to the accumulator
A: accumulator
DPTR: Data Pointer
DPTR: Data Pointer
Description: Instruction first adds the 16-bit DPTR register to the accumulator. The result of addition is then used as a memory address from which the 8-bit data is moved to the accumulator.
Syntax: MOVC A,@A+DPTR;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
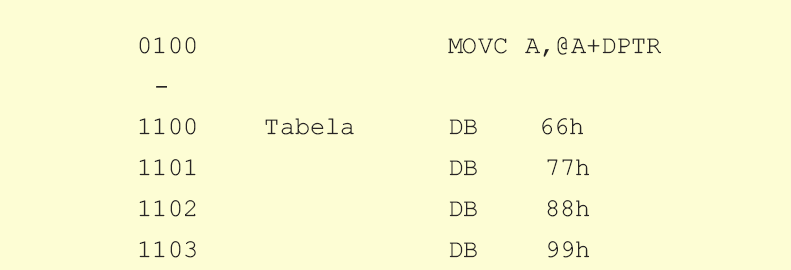
Before execution:
DPTR=1000:
A=0
A=1
A=2
A=3
DPTR=1000:
A=0
A=1
A=2
A=3
After execution:
A=66h
A=77h
A=88h
A=99h
A=66h
A=77h
A=88h
A=99h
Note: DB (Define Byte) is a directive in assembly language used to define constant.
MOV DPTR,#data16 - Loads the data pointer with a 16-bit constant
Data: constant in the range of 0-65535 (0-FFFFh)
DPTR: Data Pointer
DPTR: Data Pointer
Description: Instruction stores a 16-bit constant to the DPTR register. The 8 high bits of the constant are stored in the DPH register, while the 8 low bits are stored in the DPL register.
Syntax: MOV DPTR,#data;
Bytes: 3 (instruction code, constant (15-8), constant (7-0));
STATUS register flags: No flags affected;
Bytes: 3 (instruction code, constant (15-8), constant (7-0));
STATUS register flags: No flags affected;
EXAMPLE:

After execution: DPH=12h DPL=34h
MOVX A,@Ri - Moves the external RAM (8-bit address) to the accumulator
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: Instruction reads the content of a register in external RAM and moves it to the accumulator. The register address is stored in the Ri register (R0 or R1).
Syntax: MOVX A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
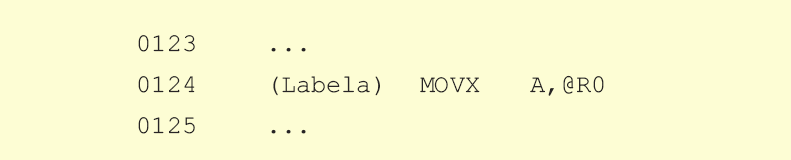
Register Address: SUM=12h
Before execution: SUM=58h R0=12h
After execution: A=58h
Before execution: SUM=58h R0=12h
After execution: A=58h
Note:
SUM Register is stored in external RAM which is 256 bytes in size.
SUM Register is stored in external RAM which is 256 bytes in size.
MOVC A,@A+PC - Moves the code byte relative to the PC to the accumulator
A: accumulator
PC: Program Counter
PC: Program Counter
Description: Instruction first adds the 16-bit PC register to the accumulator (the current program address is stored in the PC register). The result of addition is then used as a memory address from which the 8-bit data is moved to the accumulator.
Syntax: MOVC A,@A+PC;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
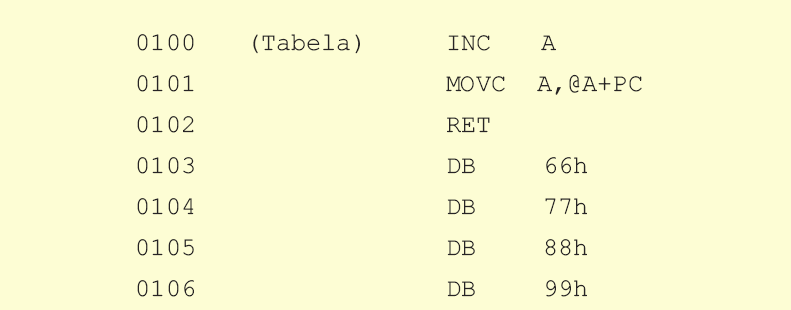
After the subroutine "Table" has been executed, one of four values is stored in the accumulator:
Before execution:
A=0
A=1
A=2
A=3
A=0
A=1
A=2
A=3
After execution:
A=66h
A=77h
A=88h
A=99h
A=66h
A=77h
A=88h
A=99h
Note: DB (Define Byte) is a directive in assembly language used to define constant.
MOVX @Ri,A - Moves the accumulator to the external RAM (8-bit address)
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: Instruction moves the accumulator to a register stored in external RAM. Its address is stored in the Ri register.
Syntax: MOVX @Ri,A;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
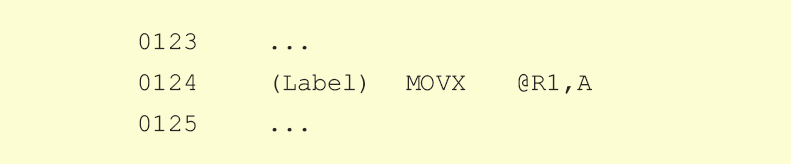
Register address: SUM=34h
Before execution: A=58 R1=34h
After execution: SUM=58h
Before execution: A=58 R1=34h
After execution: SUM=58h
NOTE:
Register SUM is located in external RAM which is 256 bytes in size.
Register SUM is located in external RAM which is 256 bytes in size.
MOVX A,@DPTR - Moves the external memory (16-bit address) to the accumulator
A: accumulator
DPRTR: Data Pointer
DPRTR: Data Pointer
Description: Instruction moves the content of a register in external memory to the accumulator. The 16-bit address of the register is stored in the DPTR register (DPH and DPL).
Syntax: MOVX A,@DPTR;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:

Register address: SUM=1234h
Before execution: DPTR=1234h SUM=58
After execution: A=58h
Before execution: DPTR=1234h SUM=58
After execution: A=58h
Note:
Register SUM is located in external RAM which is up to 64K in size.
Register SUM is located in external RAM which is up to 64K in size.
MUL AB - Multiplies A and B
A: accumulator
B: Register B
B: Register B
Description: Instruction multiplies the value in the accumulator with the value in the B register. The low-order byte of the 16-bit result is stored in the accumulator, while the high byte remains in the B register. If the result is larger than 255, the overflow flag is set. The carry flag is not affected.
Syntax: MUL AB;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:

Before execution: A=80 (50h) B=160 (A0h)
After execution: A=0 B=32h
A·B=80·160=12800 (3200h)
After execution: A=0 B=32h
A·B=80·160=12800 (3200h)
MOVX @DPTR,A - Moves the accumulator to the external RAM (16-bit address)
A: accumulator
DPTR: Data Pointer
DPTR: Data Pointer
Description: Instruction moves the accumulator to a register stored in external RAM. The 16-bit address of the register is stored in the DPTR register (DPH and DPL).
Syntax: MOVX @DPTR,A;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:

Register address: SUM=1234h
Before execution: A=58 DPTR=1234h
After execution: SUM=58h
Before execution: A=58 DPTR=1234h
After execution: SUM=58h
Note:
Register SUM is located in RAM which is up to 64K in size.
Register SUM is located in RAM which is up to 64K in size.
ORL A,Rn - OR register to the accumulator
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Description: Instruction performs logic OR operation between the accumulator and Rn register. The result is stored in the accumulator.
Syntax: ORL A,Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
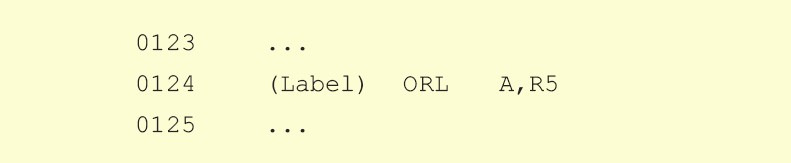
Before execution: A= C3h (11000011 Bin.)
R5= 55h (01010101 Bin.)
After execution: A= D7h (11010111 Bin.)
R5= 55h (01010101 Bin.)
After execution: A= D7h (11010111 Bin.)
NOP - No operation
Description: Instruction doesn’t perform any operation and is used when additional time delays are needed.
Syntax: NOP;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
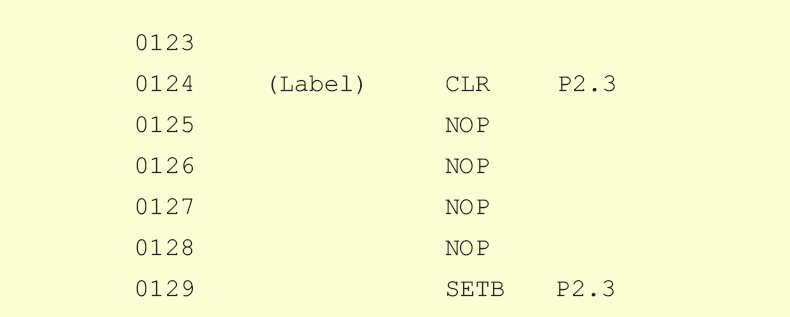
Such a sequence provides a negative pulse which lasts exactly 5 machine cycles on the P2.3. If a 12 MHz quartz crystal is used then 1 cycle lasts 1uS, which means that this output will be a low-going output pulse for 5 uS.
ORL A,@Ri - OR indirect RAM to the accumulator
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: Instruction performs logic OR operation between the accumulator and a register. As it is indirect addressing, the register address is stored in the Ri register (R0 or R1). The result is stored in the accumulator.
Syntax: ANL A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
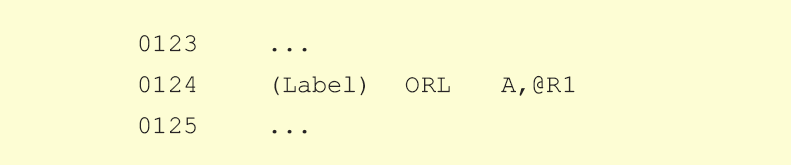
Register address: TEMP=FAh
Before execution: R1=FAh
TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
Before execution: R1=FAh
TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
ORL A,direct - OR direct byte to the accumulator
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs logic OR operation between the accumulator and a register. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the accumulator.
Syntax: ORL A,direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
EXAMPLE:
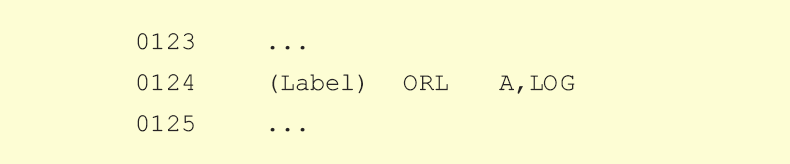
Before execution: A= C2h (11000010 Bin.)
LOG= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
LOG= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
ORL direct,A - OR accumulator to the direct byte
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs logic OR operation between a register and accumulator. As it is direct addressing, the register can be any SFRs or general- purpose register with address 0-7Fh (0-127 dec.). The result is stored in the register.
Syntax: ORL [register address], A;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
EXAMPLE:
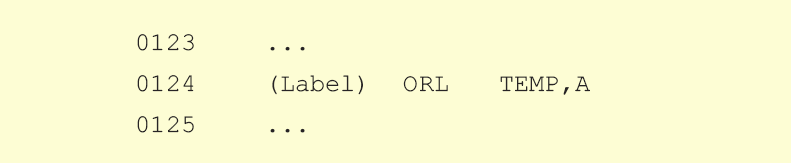
Before execution: TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
A= 54h (01010100 Bin.)
After execution: A= D6h (11010110 Bin.)
ORL A,#data - OR immediate data to the accumulator
Data: constant in the range of 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs logic OR operation between the accumulator and the immediate data. The result is stored in the accumulator.
Syntax: ORL A, #data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags affected;
EXAMPLE:
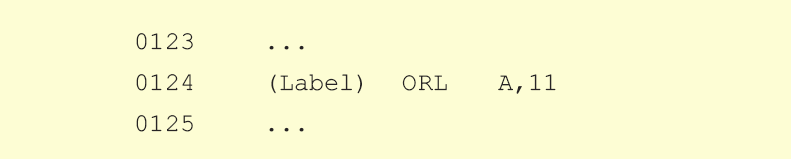
Before execution: A= C2h (11000010 Bin.)
After execution: A= C3h (11000011 Bin.)
After execution: A= C3h (11000011 Bin.)
ORL C,bit - OR direct bit to the carry flag
C: Carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction performs logic OR operation between the direct bit and the carry flag. The result is stored in the carry flag.
Syntax: ORL C,bit;
Bytes: 2 (instruction code, direct bit address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct bit address);
STATUS register flags: No flags affected;
EXAMPLE:
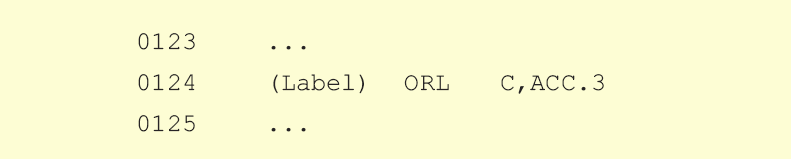
Before execution: ACC= C6h (11001010 Bin.)
C=0
After execution: C=1
C=0
After execution: C=1
ORL direct,#data - OR immediate data to direct byte
Direct: arbitrary register with address 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Description: Instruction performs logic OR operation between the immediate data and the direct byte. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the direct byte.
Syntax: ORL [register address],#data;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags affected;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags affected;
EXAMPLE:

Before execution: TEMP= C2h (11000010 Bin.)
After execution: A= D2h (11010010 Bin.)
After execution: A= D2h (11010010 Bin.)
POP direct - Pop the direct byte from the stack
Direct: arbitrary register with address 0-255 (0-FFh)
Description: Instruction first reads data from the location being pointed to by the Stack. The data is then copied to the direct byte and the value of the Stack Pointer is decremented by 1. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.).
Syntax: POP direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
EXAMPLE:
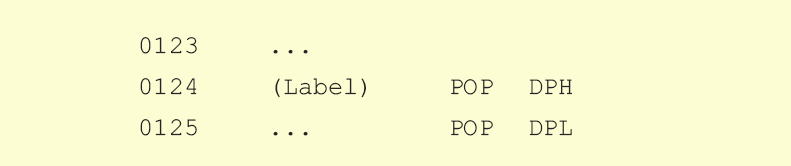
Before execution: Address Value
030h 20h
031h 23h
SP==> 032h 01h
DPTR=0123h (DPH=01, DPL=23h)
030h 20h
031h 23h
SP==> 032h 01h
DPTR=0123h (DPH=01, DPL=23h)
After execution: Address Value
SP==> 030h 20h
031h 23h
032h 01h
SP==> 030h 20h
031h 23h
032h 01h
ORL C,/bit - OR complements of direct bit to the carry flag
C: carry flag
Bit: any bit of RAM
Bit: any bit of RAM
Description: Instruction performs logic OR operation between the addressed inverted bit and the carry flag. The result is stored in the carry flag.
BIT | BIT | C | C AND BIT |
---|---|---|---|
0 | 1 | 0 | 0 |
0 | 1 | 1 | 1 |
1 | 0 | 0 | 0 |
1 | 0 | 1 | 0 |
Syntax: ORL C,/bit;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags affected;
EXAMPLE:
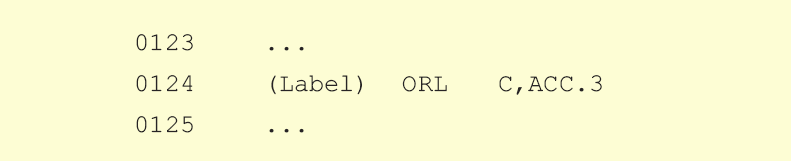
Before execution: ACC= C6h (11001010 Bin.)
C=0
After execution: C=0
C=0
After execution: C=0
RET - Return from subroutine
Description: This instruction ends every subroutine. After execution, the program proceeds with the instruction following an ACALL or LCALL.
Syntax: RET;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
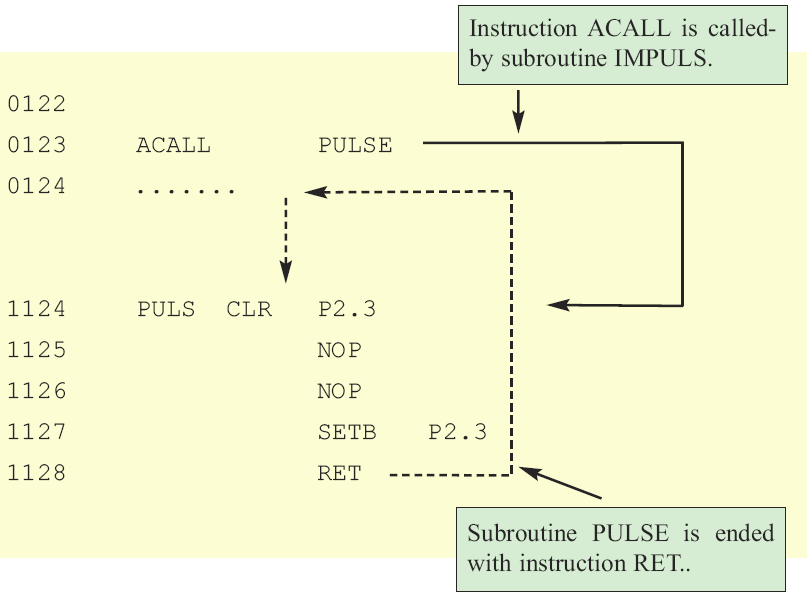
PUSH direct - Pushes the direct byte onto the stack
Data: Arbitrary register with address 0-255 (0-FFh)
Description: Address currently pointed to by the Stack Pointer is first incremented by 1 and afterwards the data from the register Rx is copied to it. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.)
Syntax: PUSH direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
EXAMPLE:

Before execution: Address Value
SP==> 030h 20h
DPTR=0123h (DPH=01, DPL=23h)
SP==> 030h 20h
DPTR=0123h (DPH=01, DPL=23h)
After execution: Address Value
030h 20h
031h 23h
SP==> 032h 01h
030h 20h
031h 23h
SP==> 032h 01h
RL A - Rotates the accumulator one bit left
A: accumulator
Description: Eight bits in the accumulator are rotated one bit left, so that the bit 7 is rotated into the bit 0 position.
Syntax: RL A;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
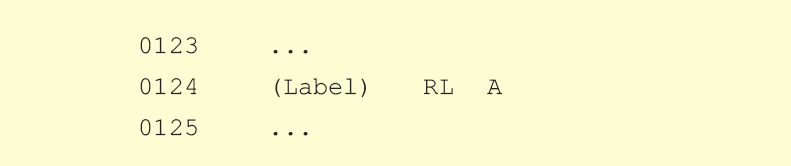
Before execution: A= C2h (11000010 Bin.)
After execution: A=85h (10000101 Bin.)
After execution: A=85h (10000101 Bin.)
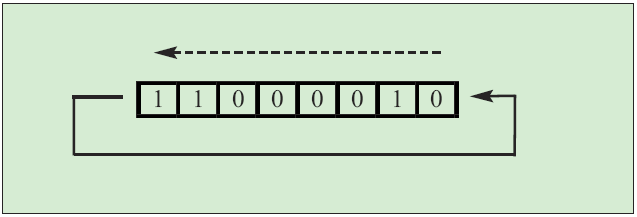
RETI - Return from interrupt
Description: This instruction ends every interrupt routine and informs processor about it. After executing the instruction, the program proceeds from where it left off. The PSW is not automatically returned its pre-interrupt status.
Syntax: RETI;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
RR A - Rotates the accumulator one bit right
A: accumulator
Description: All eight bits in the accumulator are rotated one bit right so that the bit 0 is rotated into the bit 7 position.
Syntax: RR A;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
Byte: 1 (instruction code);
STATUS register flags: No flags affected;
EXAMPLE:
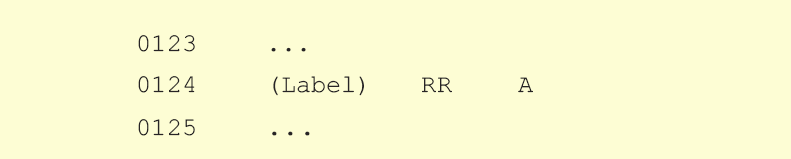
Before execution: A= C2h (11000010 Bin.)
After execution: A= 61h (01100001 Bin.)
After execution: A= 61h (01100001 Bin.)
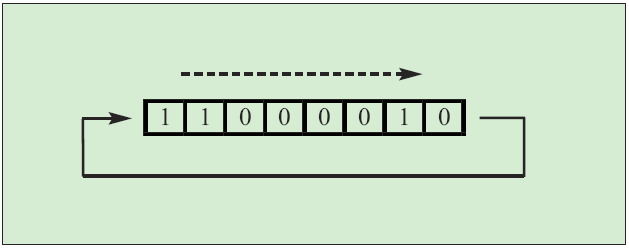
RLC A - Rotates the accumulator one bit left through the carry flag
A: accumulator
Description: All eight bits in the accumulator and carry flag are rotated one bit left. After this operation, the bit 7 is rotated into the carry flag position and the carry flag is rotated into the bit 0 position.
Syntax: RLC A;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:
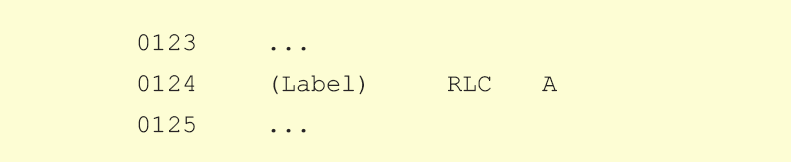
Before execution: A= C2h (11000010 Bin.)
C=0
After execution: A= 85h (10000100 Bin.)
C=1
C=0
After execution: A= 85h (10000100 Bin.)
C=1
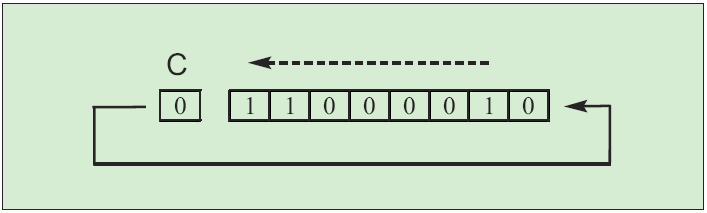
SETB C - Sets the carry flag
C: Carry flag
Description: Instruction sets the carry flag.
Syntax: SETB C;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:

After execution: C=1
RRC A - Rotates the accumulator one bit right through the carry flag
A: accumulator
Description: All eight bits in the accumulator and carry flag are rotated one bit right. After this operation, the carry flag is rotated into the bit 7 position and the bit 0 is rotated into the carry flag position.
Syntax: RRC A;
Byte: 1 (instruction code);
STATUS register flags: C;
Byte: 1 (instruction code);
STATUS register flags: C;
EXAMPLE:
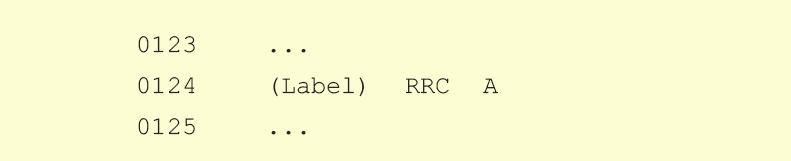
Before execution: A= C2h (11000010 Bin.)
C=0
After execution: A= 61h (01100001 Bin.)
C=0
C=0
After execution: A= 61h (01100001 Bin.)
C=0
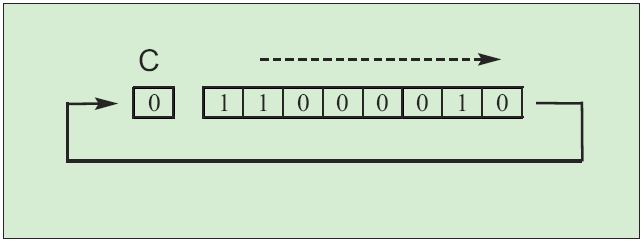
SJMP rel - Short Jump (relative address)
addr: Jump Address
Description: Instruction enables jump to the new address which should be in the range of -128 to +127 locations relative to the first following instruction.
Syntax: SJMP [jump address];
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, jump value);
STATUS register flags: No flags are affected;
EXAMPLE:
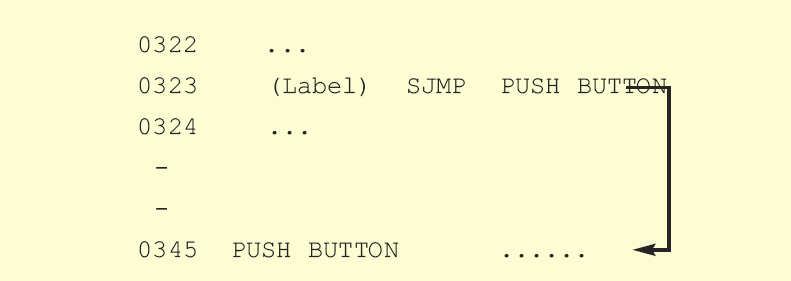
Before execution: PC=323
After execution: PC=345
After execution: PC=345
SETB bit - Sets the direct bit
Bit: any bit of RAM
Description: Instruction sets the specified bit. The register containing that bit must belong to the group of the so called bit addressable registers.
Syntax: SETB [bit address];
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, bit address);
STATUS register flags: No flags affected;
EXAMPLE:

Before execution: P0.1 = 34h (00110100)
pin 1 is configured as an output
After execution: P0.1 = 35h (00110101)
pin 1 is configured as an input
pin 1 is configured as an output
After execution: P0.1 = 35h (00110101)
pin 1 is configured as an input
SUBB A,direct - Subtracts the direct byte from the accumulator with a borrow
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction subtracts the direct byte from the accumulator with a borrow. If the higher bit is subtracted from the lower bit then the carry flag is set. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh. (0-127 dec.). The result is stored in the accumulator.
Syntax: SUBB A,direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: C, OV, AC;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: C, OV, AC;
EXAMPLE:

Before execution: A=C9h, DIF=53h, C=0
After execution: A=76h, C=0
After execution: A=76h, C=0
SUBB A,Rn - Subtracts the Rn register from the accumulator with a borrow
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Description: Instruction subtracts the Rn register from the accumulator with a borrow. If the higher bit is subtracted from the lower bit then the carry flag is set. The result is stored in the accumulator.
Syntax: SUBB A,Rn;
Byte: 1 (instruction code);
STATUS register flags: C, OV, AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV, AC;
EXAMPLE:
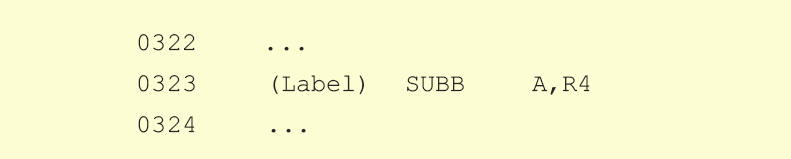
Before execution: A=C9h, R4=54h, C=1
After execution: A=74h, C=0
After execution: A=74h, C=0
Note:
The result is different (C9 - 54=75) because the carry flag is set (C=1) before the instruction starts execution.
The result is different (C9 - 54=75) because the carry flag is set (C=1) before the instruction starts execution.
SUBB A,#data - Subtracts the immediate data from the accumulator with a borrow
A: accumulator
Data: constant in the range of 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Description: Instruction subtracts the immediate data from the accumulator with a borrow. If the higher bit is subtracted from the lower bit then the carry flag is set. The result is stored in the accumulator.
Syntax: SUBB A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV, AC;
Bytes: 2 (instruction code, data);
STATUS register flags: C, OV, AC;
EXAMPLE:
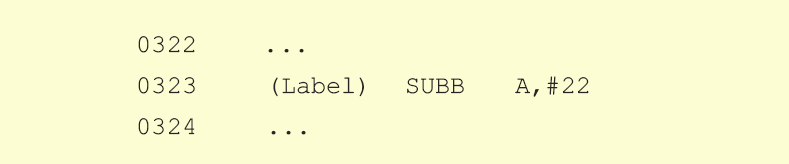
Before execution: A=C9h, C=0
After execution: A=A7h, C=0
After execution: A=A7h, C=0
SUBB A,@Ri - Subtracts the indirect RAM from the accumulator with a borrow
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: Instruction subtracts the indirectly addressed register of RAM from the accumulator with a borrow. If the higher bit is subtracted from the lower bit then the carry flag is set. As it is indirect addressing, the register address is stored in the Ri register (R0 or R1). The result is stored in the accumulator.
Syntax: SUBB A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: C, OV, AC;
Byte: 1 (instruction code);
STATUS register flags: C, OV, AC;
EXAMPLE:
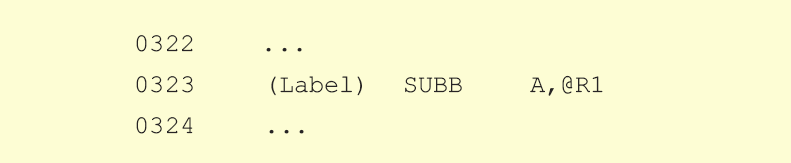
Register address: MIN=F4
Before execution: A=C9h, R1=F4h, MIN=04, C=0
After execution: A=C5h, C=0
Before execution: A=C9h, R1=F4h, MIN=04, C=0
After execution: A=C5h, C=0
XCH A,Rn - Exchanges the Rn register with the accumulator
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Description: Instruction causes the accumulator and Rn register to exchange data. The content of the accumulator is moved to the Rn register and vice versa.
Syntax: XCH A,Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
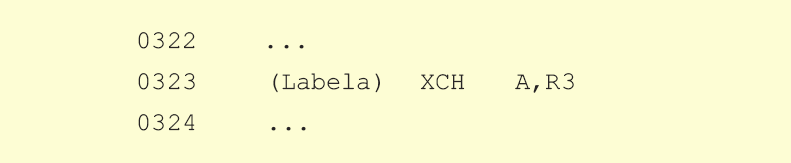
Before execution: A=C6h, R3=29h
After execution: R3=C6h, A=29h
After execution: R3=C6h, A=29h
SWAP A - Swaps nibbles within the accumulator
A: accumulator
Description: A nibble refers to a group of 4 bits within one register (bit0-bit3 and bit4-bit7). This instruction interchanges high and low nibbles of the accumulator.
Syntax: SWAP A;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:

Before execution: A=E1h (11100001)bin.
After execution: A=1Eh (00011110)bin.
After execution: A=1Eh (00011110)bin.
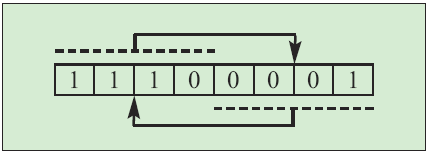
XCH A,@Ri - Exchanges the indirect RAM with the accumulator
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: Instruction moves the contents of accumulator to the indirectly addressed register of RAM and vice versa. As it is indirect addressing, the register address is stored in the register Ri (R0 or R1).
Syntax: XCH A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:

Register address: SUM=E3
Before execution: R0=E3, SUM=29h, A=98h
After execution: A=29h, SUM=98h
Before execution: R0=E3, SUM=29h, A=98h
After execution: A=29h, SUM=98h
XCH A,direct - Exchanges the direct byte with the accumulator
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction moves the contents of the accumulator into the direct byte and vice versa. As it is direct addressing, the direct byte can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.).
Syntax: XCH A,direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:
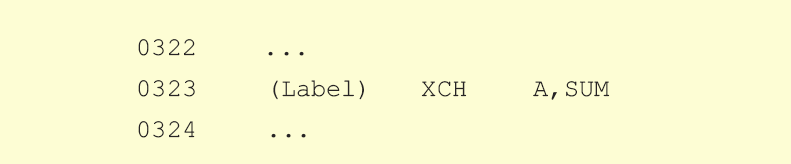
Before execution: A=FFh, SUM=29h
After execution: SUM=FFh A=29h
After execution: SUM=FFh A=29h
XRL A,Rn - Exclusive OR register to accumulator
Rn: any R register (R0-R7)
A: accumulator
A: accumulator
Description: Instruction performs exclusive OR operation between the accumulator and the Rn register. The result is stored in the accumulator.
Syntax: XRL A,Rn;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
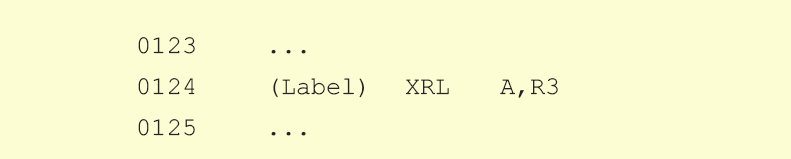
Before execution: A= C3h (11000011 Bin.)
R3= 55h (01010101 Bin.)
After execution: A= 96h (10010110 Bin.)
R3= 55h (01010101 Bin.)
After execution: A= 96h (10010110 Bin.)
XCHD A,@Ri - Exchanges the low-order nibble indirect RAM with the accumulator
Ri: register R0 or R1
A: accumulator
A: accumulator
Description: This instruction interchanges the low-order nibbles (bits 0-3) of the accumulator with the low-order nibbles of the indirectly addressed register of RAM. High-order nibbles of the accumulator and register are not affected. This instruction is mainly used when operating with BCD values. As it is indirect addressing, the register address is stored in the register Ri (R0 or R1).
Syntax: XCHD A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
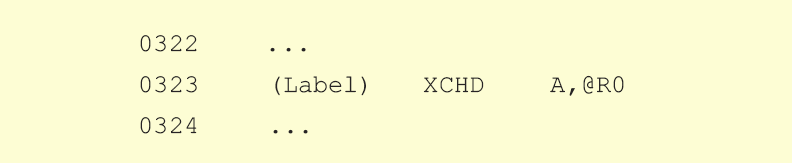
Register address: SUM=E3
Before execution: R0=E3 SUM=29h A=A8h,
After execution: A=A9h, SUM=28h
Before execution: R0=E3 SUM=29h A=A8h,
After execution: A=A9h, SUM=28h
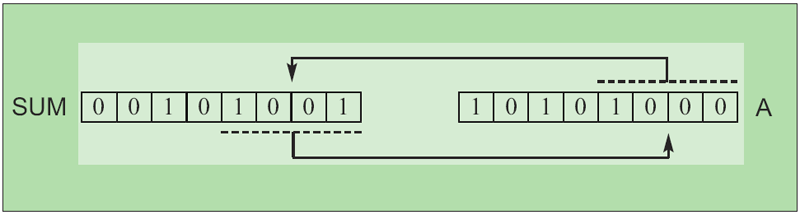
XRL A,@Ri - Exclusive OR indirect RAM to the accumulator
Ri: Register R0 or R1
A: accumulator
A: accumulator
Description: Instruction performs exclusive OR operation between the accumulator and the indirectly addressed register. As it is indirect addressing, the register address is stored in the Ri register (R0 or R1). The result is stored in the accumulator.
Syntax: XRL A,@Ri;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
Byte: 1 (instruction code);
STATUS register flags: No flags are affected;
EXAMPLE:
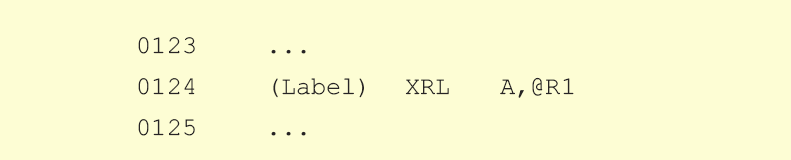
Register address: TEMP=FAh, R1=FAh
Before execution: TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
Before execution: TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
XRL A,direct - Exclusive OR direct byte to the accumulator
Direct: Arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs exclusive OR operation between the accumulator and the direct byte. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the accumulator.
Syntax: XRL A,direct;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags are affected;
EXAMPLE:

Before execution: A= C2h (11000010 Bin.)
LOG= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
LOG= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
XRL direct,A - Exclusive OR accumulator to the direct byte
Direct: arbitrary register with address 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs exclusive OR operation between the direct byte and the accumulator. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the register.
Syntax: XRL direct,A;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
Bytes: 2 (instruction code, direct byte address);
STATUS register flags: No flags affected;
EXAMPLE:
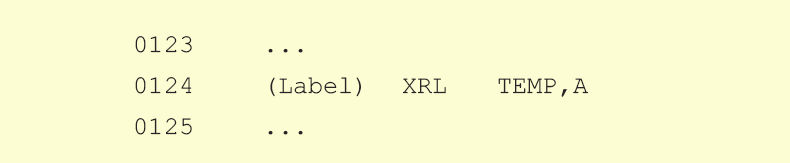
Before execution: TEMP= C2h (11000010 Bin.)
A= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
A= 54h (01010100 Bin.)
After execution: A= 96h (10010110 Bin.)
XRL A,#data - Exclusive OR immediate data to the accumulator
Data: constant in the range of 0-255 (0-FFh)
A: accumulator
A: accumulator
Description: Instruction performs exclusive OR operation between the accumulator and the immediate data. The result is stored in the accumulator.
Syntax: XRL A,#data;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
Bytes: 2 (instruction code, data);
STATUS register flags: No flags are affected;
EXAMPLE:

Before execution: A= C2h (11000010 Bin.)
X= 11h (00010001 Bin.)
After execution: A= D3h (11010011 Bin.)
X= 11h (00010001 Bin.)
After execution: A= D3h (11010011 Bin.)
XRL direct,#data - Exclusive OR immediate data to direct byte
Direct: arbitrary register with address 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Data: constant in the range of 0-255 (0-FFh)
Description: Instruction performs exclusive OR operation between the immediate data and the direct byte. As it is direct addressing, the register can be any SFRs or general-purpose register with address 0-7Fh (0-127 dec.). The result is stored in the register.
Syntax: XRL direct,#data;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags affected;
Bytes: 3 (instruction code, direct byte address, data);
STATUS register flags: No flags affected;
EXAMPLE:

Before execution: TEMP= C2h (11000010 Bin.)
X=12h (00010010 Bin.)
After execution: A= D0h (11010000 Bin.)
X=12h (00010010 Bin.)
After execution: A= D0h (11010000 Bin.)
No comments:
Post a Comment
Than 'Q'